Java Reference
In-Depth Information
How It Works
All the work is done in the
for
loop. The loop counter is
i
, and this is declared and initialized within
the
for
loop statement. The syntax of the
for
loop is shown in the following diagram:
As you see, there are three elements that control the operation of a
for
loop, and they appear between
the parentheses that follow the keyword
for
. In sequence their purpose is to:
Set the initial conditions for the loop, particularly the loop counter
Specify the condition for the loop to continue
Increment the loop counter
They are always separated by semi-colons.
The first control element is executed when the loop is first entered. Here we declare and initialize the
loop counter
i
. Because it is declared within the loop, it will not exist outside it. If you try to output the
value of
i
after the loop with a statement such as,
System.out.println("Final value of i = " + i); // Will not work outside the loop
you will find that the program will not compile. If you need to initialize and/or declare other variables
for the loop, you can do it here by separating the declarations by commas. For example, we could write:
for (int i = 1, j = 0; i <= limit; i++) {
sum += i * j++; // Add the current value of i*j to sum
}
We initialize an additional variable
j
, and, to make the loop vaguely sensible, we have modified the
value to add the sum to
i*j++
which is the equivalent of
i*(i-1)
in this case. Note that
j
will be
incremented after the product
i*j
has been calculated. You could declare other variables here, but
note that it would not make sense to declare
sum
at this point. If you can't figure out why, delete the
original declaration of
sum
and, put it in the
for
loop instead to see what happens. The program won't
compile - right? After the loop ends the variable
sum
no longer exists, so you can't reference it.

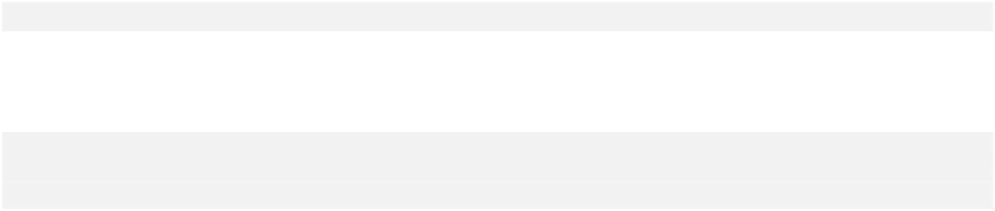






