Java Reference
In-Depth Information
System.out.println("Value is now "+value);
value *= 2;
System.out.println("Value is now "+value);
value *= 2;
System.out.println("Value is now "+value);
value *= 2;
System.out.println("Value is now "+value);
value *= 2;
System.out.println("Value is now "+value);
value *= 2;
System.out.println("Value is now "+value);
value *= 2;
System.out.println("Value is now "+value);
}
}
The same pair of statements has been entered eight times. This is a rather tedious way of doing things. If
the program for the company payroll had to include separate statements for each employee, it would
never get written. A loop removes this sort of the difficulty. We could write the method
main()
to do
the same as the code above as:
public static void main(String[] args) {
byte value = 1;
for (int i=0; i<8 ; i++) {
value *= 2;
System.out.println("Value is now " + value);
}
}
The
for
loop statement causes the statements in the following block to be repeated eight times. The
number of times it is to be repeated is determined by the stuff between parentheses following the
keyword
for
- we will see how in a moment. The point is you could, in theory, repeat the same block
of statements as many times as you want - a thousand or a million - it is just as easy and it doesn't
require any more lines of code. The primary purpose of the
for
loop is to execute a block of statements
a given number of times.
There are three kinds of loop statements you can use, so let's look at these in general terms first:
1.
The
for
loop:
for (
initialization
_
expression
;
loop
_
condition ;
increment
_
expression
) {
// statements
}
The control of the
for
loop appears in parentheses following the keyword
for
. It has three parts
separated by semi-colons.
The first part, the
initialization
_
expression
, is executed before execution of the loop starts.
This is typically used to initialize a counter for the number of loop iterations - for example i = 0. With a
loop controlled by a counter, you can count up or down using an integer or a floating point variable.
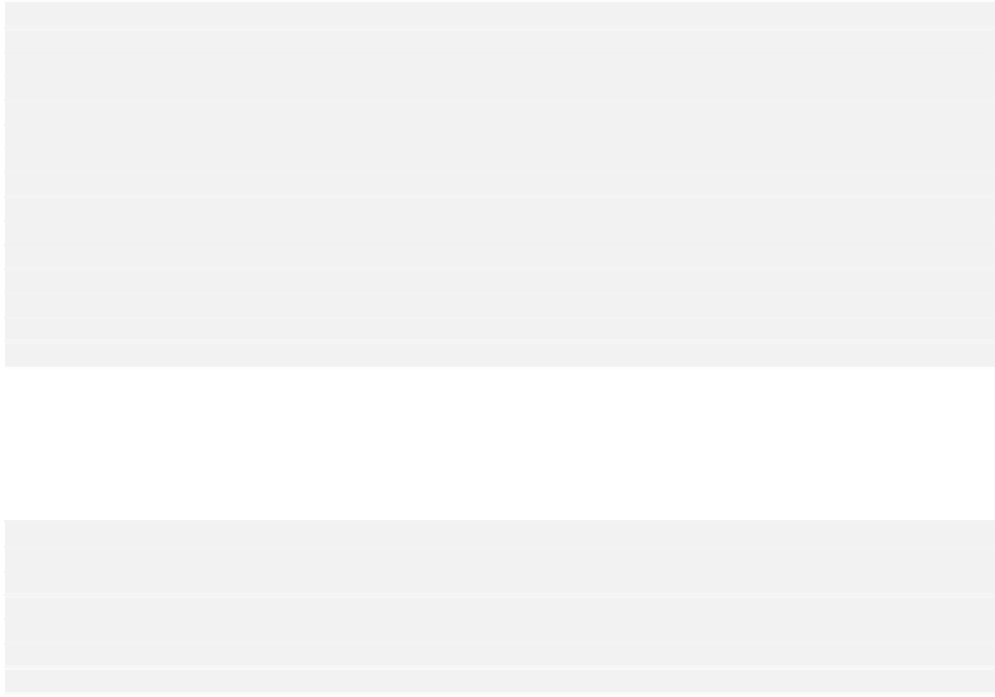








