Java Reference
In-Depth Information
Here the variable
yesNo
receives a character from the keyboard somehow. You want a different action
depending on whether the user enters '
Y
' or '
N
' but you want to be able to accept either uppercase or
lowercase entries. This
switch
does just this by putting the case labels together. Note that there is no
default case here. If
yesNo
contains a character other than those identified in the case statements, the
switch statement has no effect. You might add a default case in this kind of situation to output a message
to indicate that the value in
yesNo
is not valid.
Of course, you could also implement this logic using
if
statements:
if(yesNo=='n' || yesNo=='N') {
System.out.println("No selected");
} else {
if(yesNo=='y' || yesNo=='Y') {
System.out.println("Yes selected");
}
}
I prefer the
switch
statement as I think it's easier to follow, but you decide for yourself.
Variable Scope
The
scope
of a variable is the part of the program over which the variable name can be referenced - in
other words, where you can use the variable in the program. Every variable that we have declared so far
in program examples has been defined within the context of a method, the method
main()
. Variables
that are declared within a method are called
local variables
, as they are only accessible within the
confines of the method in which they are declared. However, they are not necessarily accessible
everywhere in the code for the method in which they are declared. Look at the example in the
illustration below that shows nested blocks inside a method.
{
int a = 1; // Declare and define a
// Reference to a is OK here
// Reference to b here is an error
{
// Reference to a here is OK
// Reference to b here is still an error
int b = 2; // Declare and define b
// References to a and b are OK here - b exists now
}
// Reference to b is an error here - it doesn't exist
// Reference to a is still OK though
}
A variable does not exist before its declaration; you can only refer to it after it has been declared. It
continues to exist until the end of the block in which it is defined, and that includes any blocks nested
within the block containing its declaration. The variable
b
only exists within the inner block. After the
brace at the end of the inner block,
b
no longer exists so you can't refer to it. The variable
a
is still
around though since it survives until the last brace.



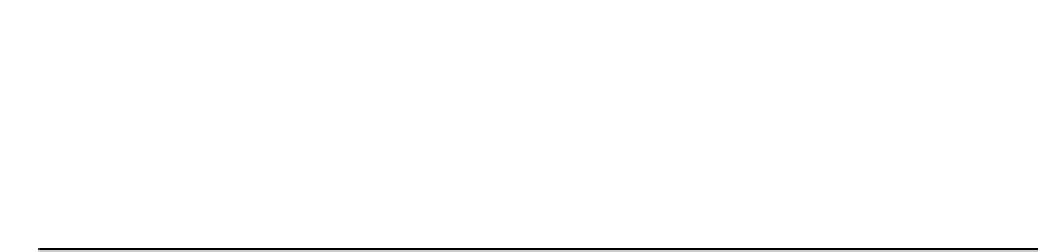