Java Reference
In-Depth Information
public void addElementNode(Document doc) {
org.w3c.dom.Element rectElement = doc.createElement("rectangle");
// Create the angle attribute and attach it to the <rectangle> node
Attr attr = doc.createAttribute("angle");
attr.setValue(String.valueOf(angle));
rectElement.setAttributeNode(attr);
// Append the <color>, <position>, and <bottomright> nodes as children
rectElement.appendChild(createColorElement(doc));
rectElement.appendChild(createPositionElement(doc));
rectElement.appendChild(createBottomrightElement(doc));
doc.getDocumentElement().appendChild(rectElement);
}
We also must define the
createBottomrightElement()
method in the
Element.Rectangle
class:
private org.w3c.dom.Element createBottomrightElement(Document doc) {
return createPointTypeElement(doc, "bottomright",
String.valueOf(rectangle.width+position.x),
String.valueOf(rectangle.height+position.y));
}
A rectangle is defined relative to the origin so we have to adjust the coordinates of the bottom right
corner by adding the corresponding
position
coordinates.
Adding a Circle Node
Creating the node for a
<circle>
element is not very different:
public void addElementNode(Document doc) {
org.w3c.dom.Element circleElement = doc.createElement("circle");
// Create the radius attribute and attach it to the <circle> node
Attr attr = doc.createAttribute("radius");
attr.setValue(String.valueOf(circle.width/2.0));
circleElement.setAttributeNode(attr);
// Create the angle attribute and attach it to the <circle> node
attr = doc.createAttribute("angle");
attr.setValue(String.valueOf(angle));
circleElement.setAttributeNode(attr);
// Append the <color> and <position> nodes as children
circleElement.appendChild(createColorElement(doc));
circleElement.appendChild(createPositionElement(doc));
doc.getDocumentElement().appendChild(circleElement);
}
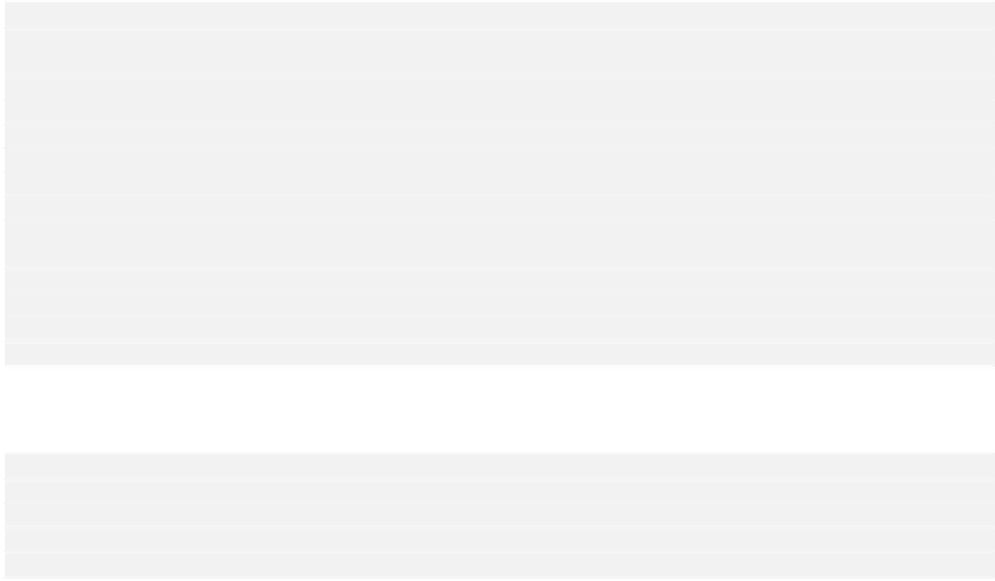








