Java Reference
In-Depth Information
Similarly, calling the
setValidating()
method with an argument of
true
will cause the factory
object to produce parsers that will validate the XML as a document is parsed. A validating parser will
verify that the document body has a DTD and that the document content is consistent with the DTD
and any internal subset that is included in the
DOCTYPE
declaration. Of course, if you configure the
factory object to create a parser that is either namespace aware or validating, the parser you intend to
use must include the capability, otherwise a request to create a parser will fail.
We can now use our
SAXParserFactory
object to create a
SAXParser
object as follows:
SAXParser parser = null;
try {
parser = spf.newSAXParser();
}catch(SAXException e){
e.printStackTrace(System.err);
System.exit(1);
} catch(ParserConfigurationException e) {
e.printStackTrace(System.err);
System.exit(1);
}
The
SAXParser
object that is created here will encapsulate the default parser. The
newSAXParser()
method for the factory object can throw the two exceptions we are catching here. A
ParserConfigurationException
will be thrown if a parser cannot be created consistent with the
configuration determined by the
SAXParserFactory
object and a
SAXException
will be thrown if
any other error occurs. For instance, if you call the
setValidating()
option and the parser does not
have the capability for validating documents, this exception would be thrown. This should not arise
with the parser supplied by default, though, since it supports both of these features.
The
ParserConfigurationException
class is defined in the
javax.xml.parsers
package, but
the
SAXException
class is in the
org.xml.sax
package so we need a separate import statement for
that. Now let's see what the default parser is by putting the code fragments we have looked at so far
together in a working example.
Try It Out - Accessing a SAX Parser
Here's the code to create a
SAXParser
object and output some details about it to the command line:
import javax.xml.parsers.SAXParserFactory;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.ParserConfigurationException;
import org.xml.sax.SAXException;
public class TrySAX {
public static void main(String args[]) {
// Create factory object
SAXParserFactory spf = SAXParserFactory.newInstance();
System.out.println("Parser will "+(spf.isNamespaceAware()?"":"not ") +
"be namespace aware");
System.out.println("Parser will "+(spf.isValidating()?"":"not ") +
"validate XML");
SAXParser parser = null; // Stores parser reference
try {
parser = spf.newSAXParser(); // Create parser object
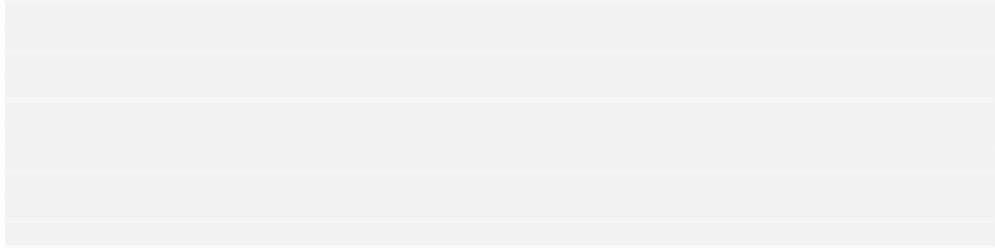









