Cryptography Reference
In-Depth Information
The rest of the code to implement the P-box is not too bad. We have to do an ugly calculation that, for each
bit position in the P-box, determines if that input bit is set. If so, then it determines which bit the set bit maps to
in the output and sets it as well. All bits not set in this way are set to zero.
The next piece of code (
Listing 4-2
)
is used for splitting apart the pieces to be fed to the S-boxes and then put
back together — hence the
mux
(multiplex, or combine) and
demux
(demultiplex, or break apart) functions.
Listing 4-2
Python code for the EASY1 multiplexing and demultiplexing.
##########################################
# Takes 36-bit to six 6-bit values
# and vice-versa
##########################################
def
demux(x):
y = []
for
i
in
range(0,6):
y.append((x >> (i * 6)) & 0x3f)
return
y
def
mux(x):
y = 0l
for
i
in
range(0,6):
y = y ^ (x[i] << (i * 6))
return
y
The trickiest part of this code is the use of the bit shift operators, which simply take the left-hand side of the
expression and shift the bits left (for <<) or right (for >>) by the number on the right. The
demux
function also
uses an AND operation by
0x3F
, which is the
bit mask
representing the binary expression
111111
— that is,
six 1's, which will drop all the bits to the left, returning only the rightmost six bits.
Finally, we write one last helper function: the code to XOR in the keys. This code is shown in
Listing 4-3
—
nothing too fancy there.
Listing 4-3
Python code for EASY1 key XORing.
##########################################
# Key mixing
##########################################
def
mix(p, k):
v = []
key = demux(k)
for
i
in
range(0,6):
v.append(p[i] ^ key[i])
return
v
After all of these helper functions, we can put in the code to do the actual encryption: the round function and
a wrapper function (encrypt). This code is shown in
Listing 4-4
.
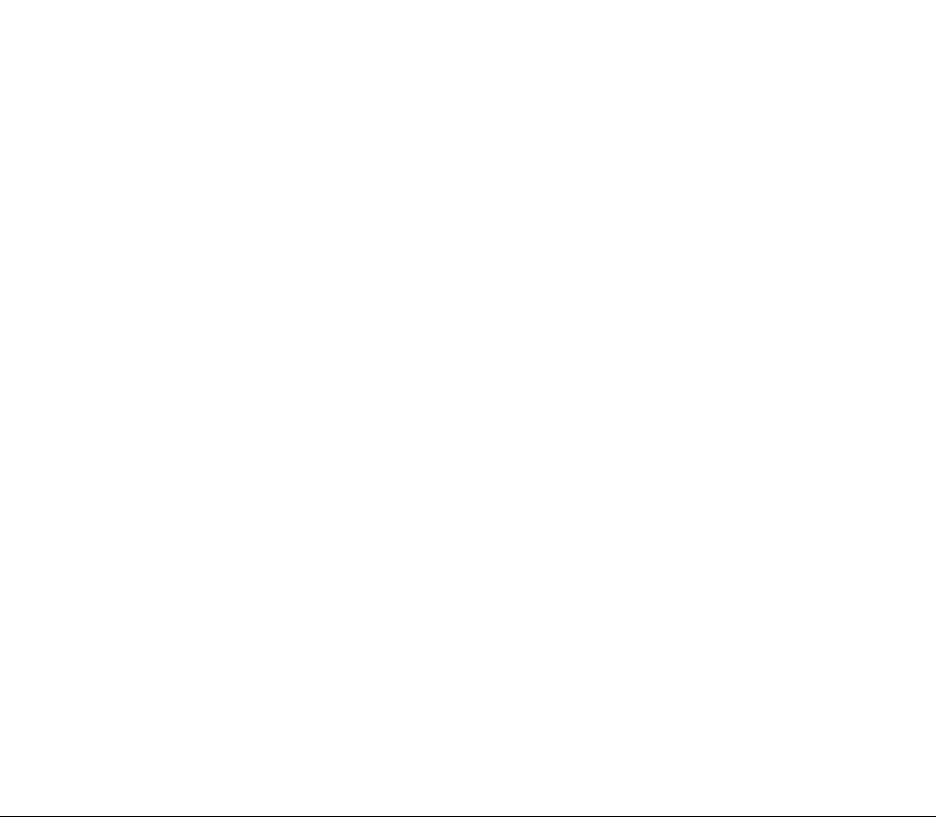



Search WWH ::

Custom Search