Cryptography Reference
In-Depth Information
At decryption time, then, you fi rst decrypt a block, and then XOR that with
the
encrypted
previous block.
But what about the fi rst block? The initialization vector must be remembered
and transmitted (or agreed upon) before decryption can continue. To support
CBC in decryption, you have to change the order of things just a bit as shown
in Listing 2-20.
Listing 2-20:
“des.c” des_operate with CBC for encrypt or decrypt
while ( input_len )
{
memcpy( ( void * ) input_block, ( void * ) input, DES_BLOCK_SIZE );
if ( operation == OP_ENCRYPT )
{
xor( input_block, iv, DES_BLOCK_SIZE ); // implement CBC
des_block_operate( input_block, output, key, operation );
memcpy( ( void * ) iv, ( void * ) output, DES_BLOCK_SIZE ); // CBC
}
if ( operation == OP_DECRYPT )
{
des_block_operate( input_block, output, key, operation );
xor( output, iv, DES_BLOCK_SIZE );
memcpy( ( void * ) iv, ( void * ) input, DES_BLOCK_SIZE ); // CBC
}
input += DES_BLOCK_SIZE;
output += DES_BLOCK_SIZE;
input_len -= DES_BLOCK_SIZE;
}
And fi nally, the decrypt routine that passes in the initialization vector and
removes the padding that was inserted, using the PKCS #5 padding scheme, is
shown in Listing 2-21.
Listing 2-21:
“des.c” des_decrypt
void des_decrypt( const unsigned char *ciphertext,
const int ciphertext_len,
unsigned char *plaintext,
const unsigned char *iv,
const unsigned char *key )
{
des_operate( ciphertext, ciphertext_len, plaintext, iv, key, OP_DECRYPT );
// Remove any padding on the end of the input
//plaintext[ ciphertext_len - plaintext[ ciphertext_len - 1 ] ] = 0x0;
}
The commented-out line at the end of listing 2-21 illustrates how you might
remove padding. As you can see, removing the padding is simple; just read the
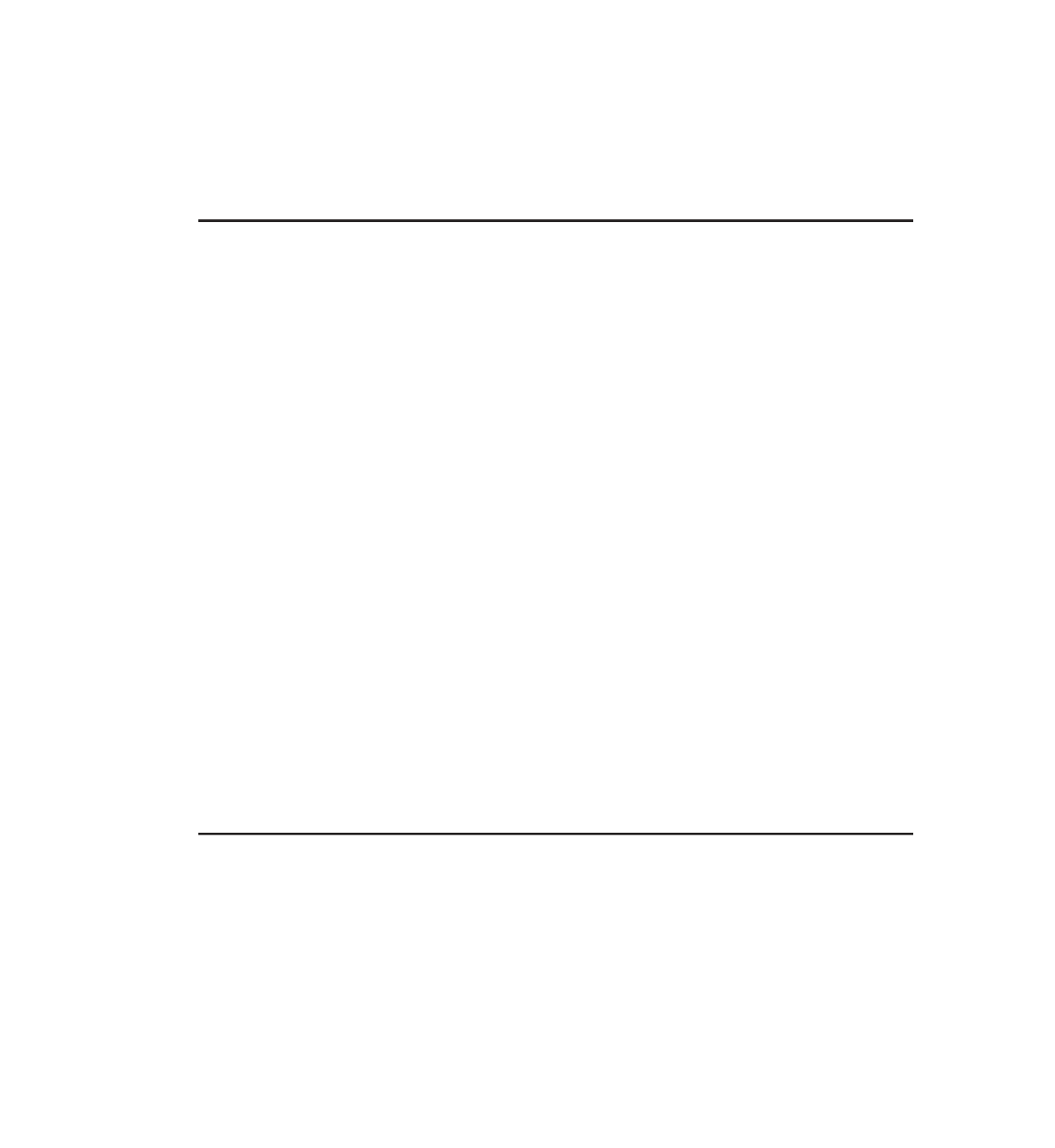



Search WWH ::

Custom Search