Cryptography Reference
In-Depth Information
free( encrypt_buf );
free( encrypted );
}
if ( send( connection, ( void * ) buffer, buf_len, 0 ) < buf_len )
{
return -1;
}
parameters->write_sequence_number++;
free( buffer );
}
As you can see, the bulk of
send_message
is now handling encryption. Check
to see if the active cipher spec requires that the message be padded to a certain
multiple:
if ( parameters->active_cipher_spec->block_size )
{
padding = parameters->active_cipher_spec->block_size -
( data_len % parameters->active_cipher_spec->block_size );
}
In practice,
block_size
is always either 8 (for DES or 3DES) or 0 (for RC4).
Next, allocate enough space for the SSLv2 header, which now is three bytes
instead of two, the MAC, the data itself, and the padding.
buf_len = 3 + // sizeof header
parameters->active_cipher_spec->hash_size + // sizeof mac
data_len + // sizeof data
padding; // sizeof padding
buffer = malloc( buf_len );
header_len = htons( buf_len - 3 );
memcpy( buffer, &header_len, 2 );
buffer[ 2 ] = padding;
encrypt_buf = malloc( buf_len - 3 );
encrypted = malloc( buf_len - 3 );
memset( encrypt_buf, '\0', buf_len - 3 );
Notice the allocation of two buffers.
buffer
is the memory array that is actu-
ally sent over the connection. This is where the three-byte header is passed.
Actually, the fi rst two bytes are the length of the message, just as they were
in the two-byte header. The third byte encodes the amount of padding on the
end of the message, which is required to be present even if the cipher spec is
a stream cipher.
NOTE
Technically, this code is wrong. The next-to-most-signifi cant bit in a
three-byte header is reserved; if bit 6 is set to 1, then the message should be
treated as a “security escape.” What this might mean and what you might do

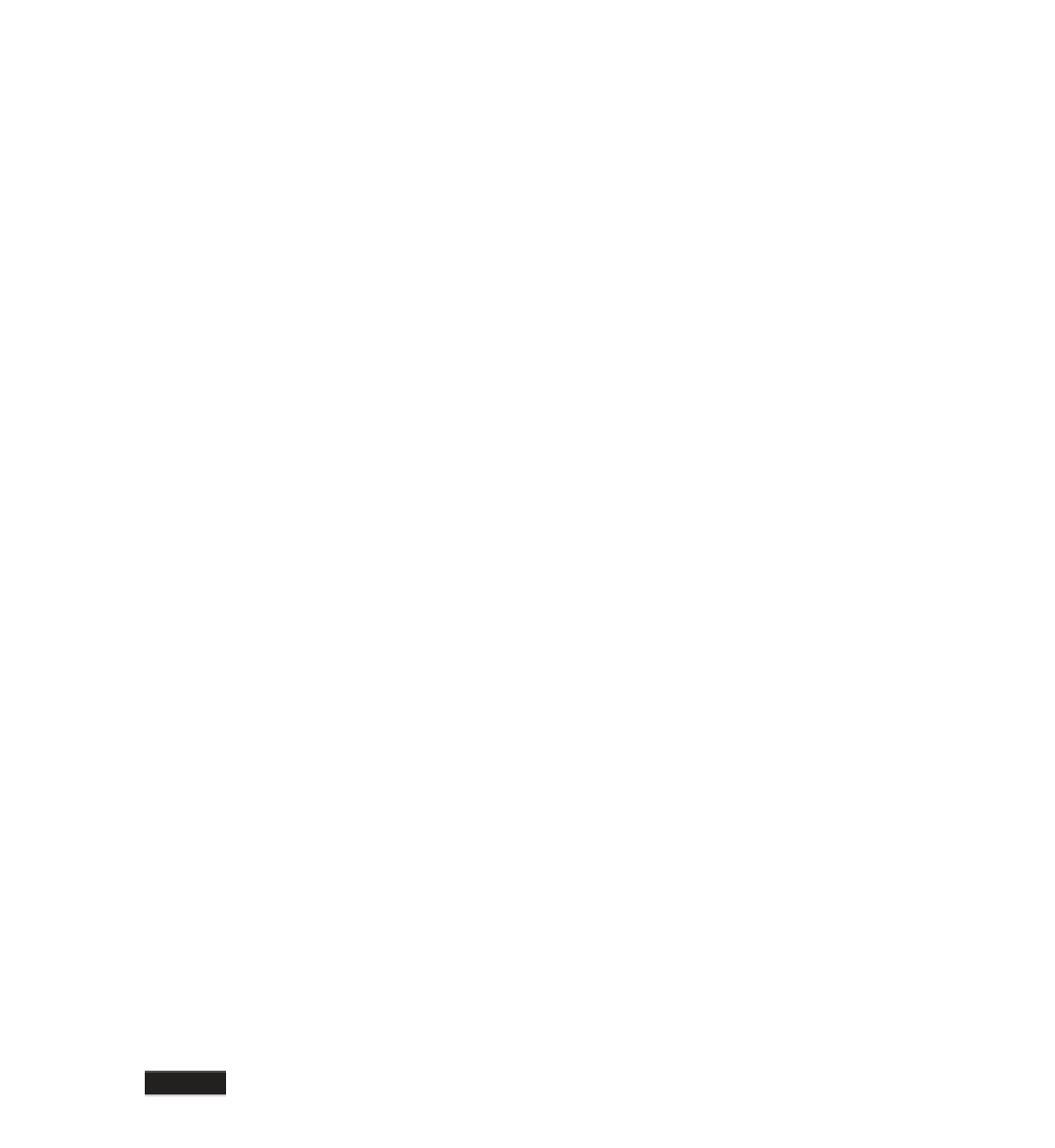



Search WWH ::

Custom Search