Cryptography Reference
In-Depth Information
const unsigned char *addldata,
const int addldata_len,
unsigned char output[],
void *iv,
const unsigned char *key )
{
return aes_gcm_process( input, input_len,
addldata, addldata_len,
output, iv, key, 0 );
}
int aes_gcm_decrypt( const unsigned char *input,
const int input_len,
const unsigned char *addldata,
const int addldata_len,
unsigned char output[],
void *iv,
const unsigned char *key )
{
return aes_gcm_process( input, input_len,
addldata, addldata_len,
output, iv, key, 1 );
}
Now modify
aes_ccm_process
and
aes_gcm_process
to accept and authen-
ticate the associated data. This is not too terribly complex; you just run the
associated data through the MAC computation before beginning the encrypt/
MAC process. The only complicating factor for CCM is that the fi rst two bytes of
the fi rst block of associated data must include the length of the additional data.
Remember that every time you MAC anything, you must include the length of
what you're MAC'ing in the processing somehow to ensure that two inputs of
differing lengths whose trailing bytes are all zeros MAC to different values (if
the reason for this isn't clear, you might want to jump back and briefl y review
Chapter 4). AES-CCM with additional data support is shown in Listing 9-21.
Listing 9-21:
“aes.c” aes_ccm_process with associated data
int aes_ccm_process( const unsigned char *input,
int input_len,
const unsigned char *addldata,
unsigned short addldata_len,
unsigned char *output,
void *iv,
const unsigned char *key,
int decrypt )
{
…
memset( input_block, '\0', AES_BLOCK_SIZE );
input_block[ 0 ] = 0x1F; // t = mac_length = 8 bytes, q = 8 bytes (so n = 7)
(Continued)
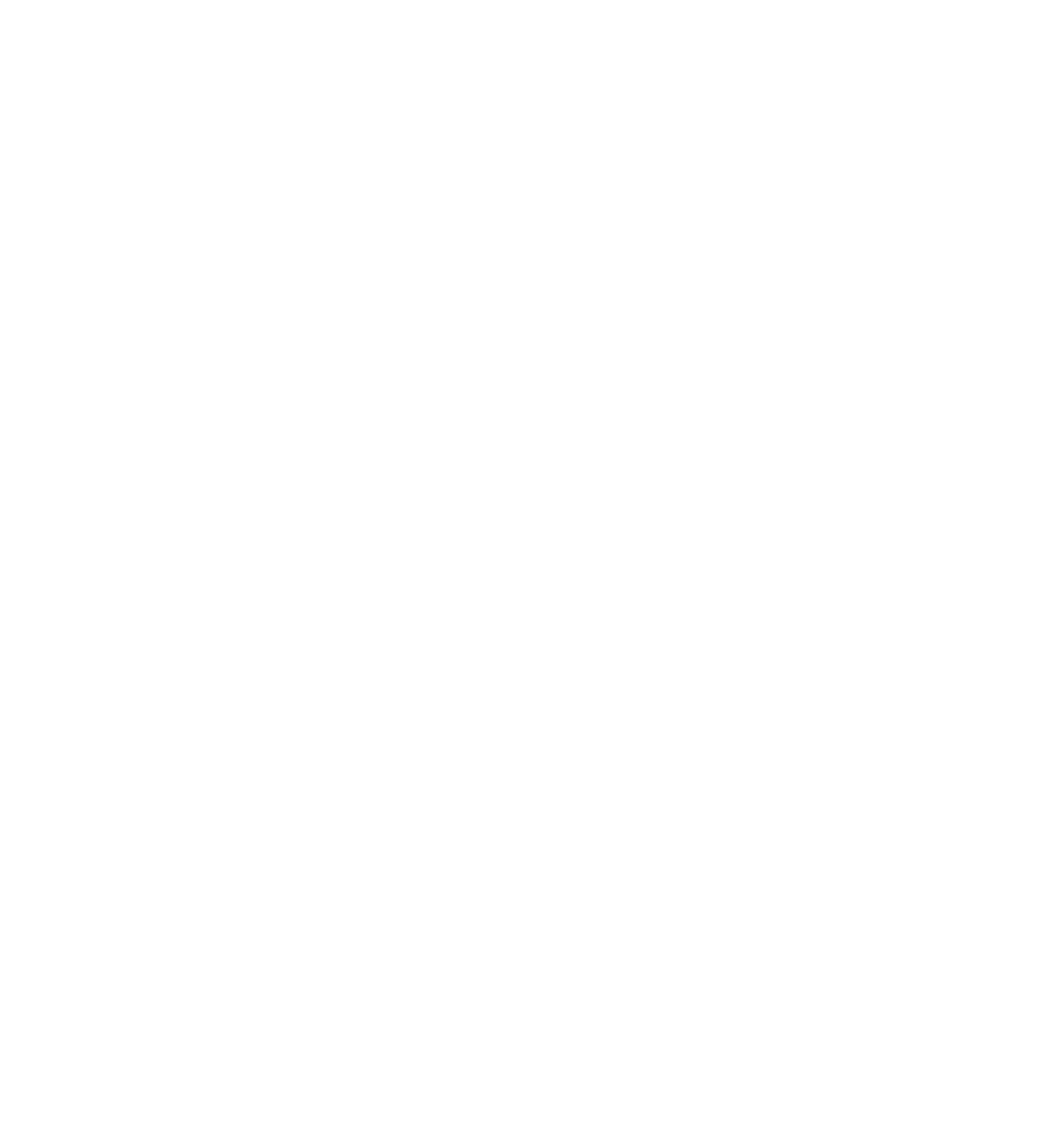




Search WWH ::

Custom Search