Cryptography Reference
In-Depth Information
Assigning a Unique Session ID to Each Session
The fi rst change you must make to support server-side session resumption
is to assign a unique session ID to each session. Recall that in Chapter 7, each
server hello message is sent with an empty session ID, which the client should
interpret as “server unable to resume session.” The changes to assign unique
session IDs to each session are shown in Listing 8-9.
Listing 8-9:
“tls.c” server-side session resumption support
static int next_session_id = 1;
static int send_server_hello( int connection, TLSParameters *parameters )
{
ServerHello package;
…
memcpy( package.random.random_bytes, parameters->server_random + 4, 28 );
if ( parameters->session_id_length == 0 )
{
// Assign a new session ID
memcpy( parameters->session_id, &next_session_id, sizeof( int ) );
parameters->session_id_length = sizeof( int );
next_session_id++;
}
package.session_id_length =
parameters->session_id_length
;
Here, I've gone ahead and made
next_session_id
a static variable. This could
potentially create some threading problems if I was using this in a multithreaded
application. The session ID in this case is a monotonically increasing 4-byte
identifi er. I also didn't bother correcting for host ordering, so if this is run on a
little-endian machine, the fi rst session ID shows up as 0x01000000, the second
as 0x02000000, and so on. This increase doesn't really matter in this case, as long
as each is unique. Finally, notice that the code checks whether a session ID has
already been assigned before assigning one. This will be the case, when session
resumption is added in Listing 8-15, if a session is being resumed.
Adding Session ID Storage
To actually store these, you need some internal data structure that can easily
map session IDs to master secret values. As a nod to effi ciency, go ahead and
make this a hash table. First, declare a storage structure and a static instance to
contain it as shown in Listing 8-10.
Listing 8-10:
“tls.c” session storage hash table
#define HASH_TABLE_SIZE 100
typedef struct StoredSessionsList_t
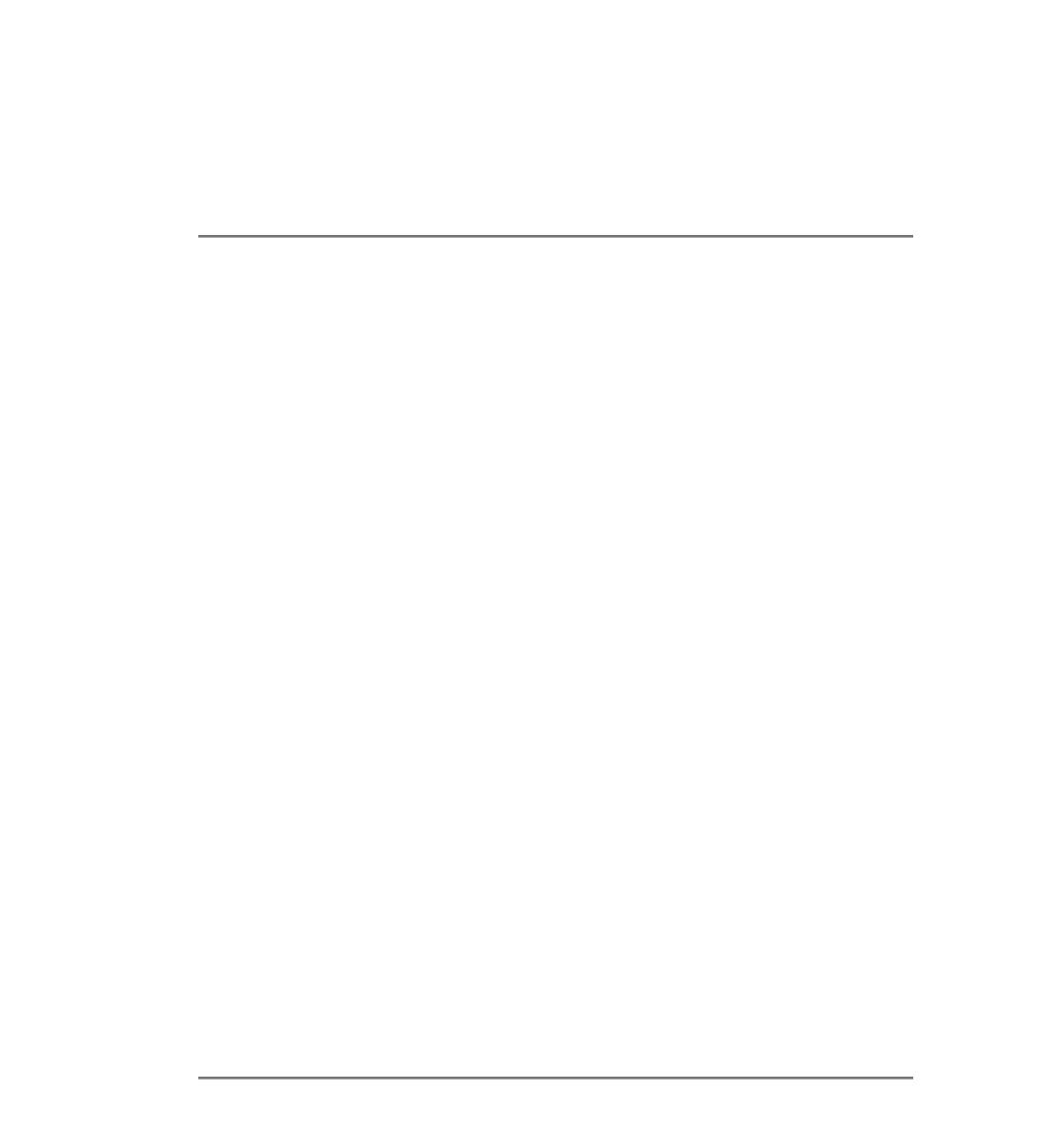



Search WWH ::

Custom Search