Cryptography Reference
In-Depth Information
You might try to get around this by changing the IV size in the cipher suite
declaration to 256 so that you'd get a state vector here. Unfortunately, this won't
work: You'd get 256 pseudo-random bytes rather than an array of 0's as
rc4_
encrypt
expects on its fi rst call. The ideal way to handle this would be to defi ne
a
cipher_init
routine that should be called on fi rst invocation. However, the
simple hack in Listing 6-65 works well enough:
Listing 6-65:
“tls.c” calculate_keys with a special RC4 exception
static void calculate_keys( TLSParameters *parameters )
{
…
switch ( suite->id )
{
case TLS_RSA_EXPORT_WITH_RC4_40_MD5:
case TLS_RSA_WITH_RC4_128_MD5:
case TLS_RSA_WITH_RC4_128_SHA:
case TLS_DH_anon_EXPORT_WITH_RC4_40_MD5:
case TLS_DH_anon_WITH_RC4_128_MD5:
{
rc4_state *read_state = malloc( sizeof( rc4_state ) );
rc4_state *write_state = malloc( sizeof( rc4_state ) );
read_state->i = read_state->j = write_state->i = write_state->j = 0;
send_parameters->IV = ( unsigned char * ) read_state;
recv_parameters->IV = ( unsigned char * ) write_state;
memset( read_state->S, '\0', RC4_STATE_ARRAY_LEN );
memset( write_state->S, '\0', RC4_STATE_ARRAY_LEN );
}
break;
default:
break;
}
free( key_block );
At this point,
send_parameters->IV
is no longer necessarily an IV, but a void
pointer to the state of the cipher suite. Although the code would be clearer if
it were renamed, the specifi cation refers specifi cally to IV in several places, so
leave it this way.
Updating Each Invocation of send_message
Of course, because you're now applying the active encryption function to every
sent message, you must also go through and update each invocation of
send_
message
to include the active
ProtectionParameters
, as in Listing 6-66.
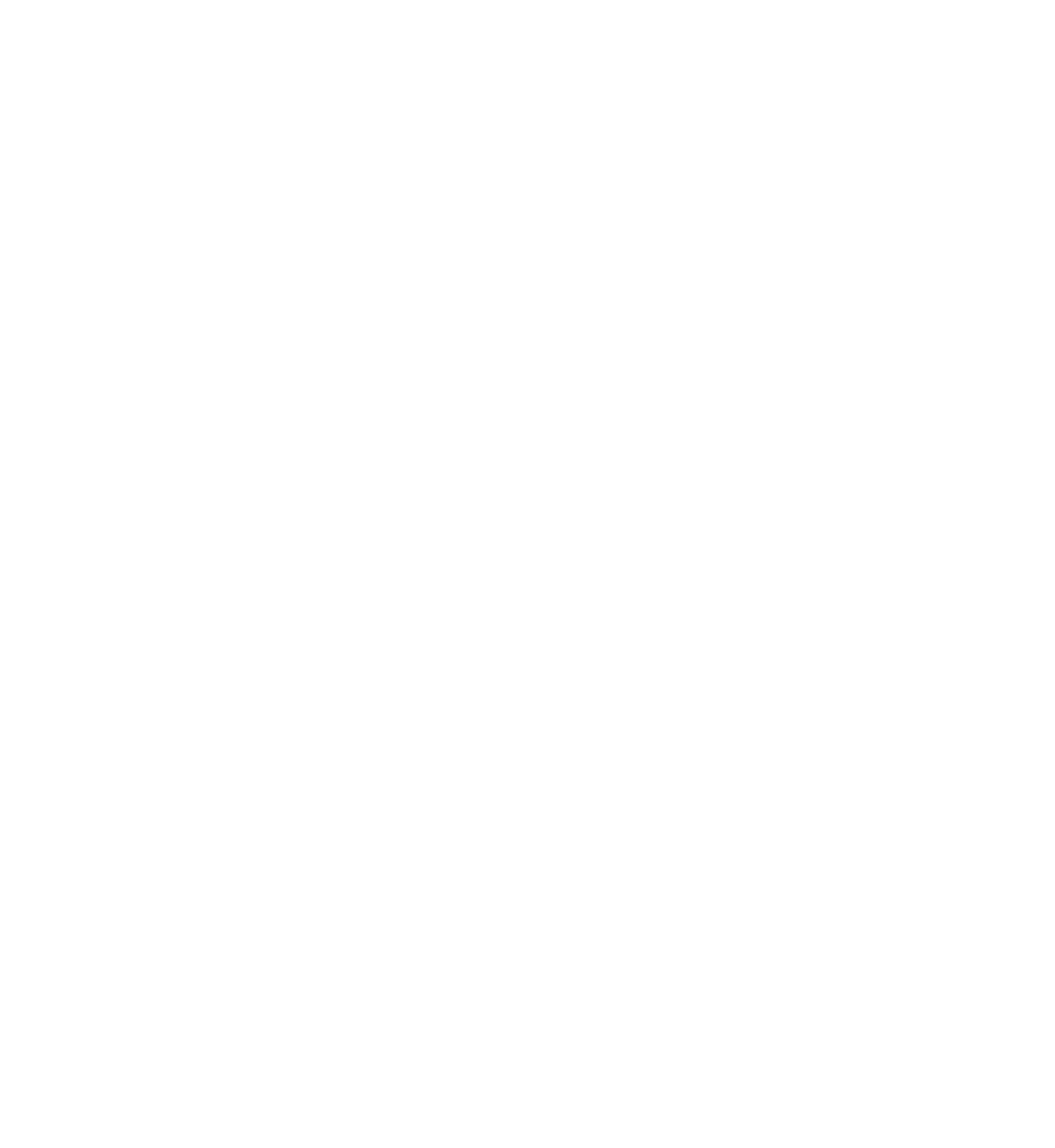




Search WWH ::

Custom Search