Cryptography Reference
In-Depth Information
divide( c, n, NULL );
free_huge( &counter );
free_huge( &one );
// Remainder (result) is now in c
}
Remember that encryption and decryption are the exact same routines, just
with the exponents switched; you can use this same routine to encrypt by pass-
ing in
e
and decrypt by passing in
d
. Just keep multiplying
m
by itself (notice
that
m
was copied into
c
once at the beginning) and incrementing a counter by
1 each time until you've done it
e
times. Finally, divide the whole mess by
n
and
the result is in
c
. Here's how you might call this:
huge e, d, n, m, c;
set_huge( &e, 79 );
set_huge( &d, 1019 );
set_huge( &n, 3337 );
set_huge( &m, 688 );
rsa_compute( &m, &e, &n, &c );
printf( “Encrypted to: %d\n”, c.rep[ 0 ] );
set_huge( &m, 0 );
rsa_compute( &c, &d, &n, &m );
printf( “Decrypted to: %d\n”, m.rep[ 0 ] );
Encrypting with RSA
Because this example uses small numbers, you can verify the accuracy by just
printing out the single
int
representing
c
and
m
:
Encrypted to: 1570
Decrypted to: 688
The encrypted representation of the number 688 is 1,570. You can decrypt
and verify that you get back what you put in.
However, this public exponent 79 is a small number for RSA, and the modu-
lus 3,337 is microscopic — if you used numbers this small, an attacker could
decipher your message using pencil and paper. Even with these small numbers,
688
79
takes up 1,356 bytes. And this is for a small
e
. For reasons you see later, a
more common
e
value is 65,537.
NOTE
Note that everybody can, and generally does, use the same
e
value as
long as the
n
— and by extension, the
d
— are different.
A 32-bit integer raised to the power of 65,537 takes up an unrealistic amount
of memory. I tried this on my computer and, after 20 minutes, I had computed

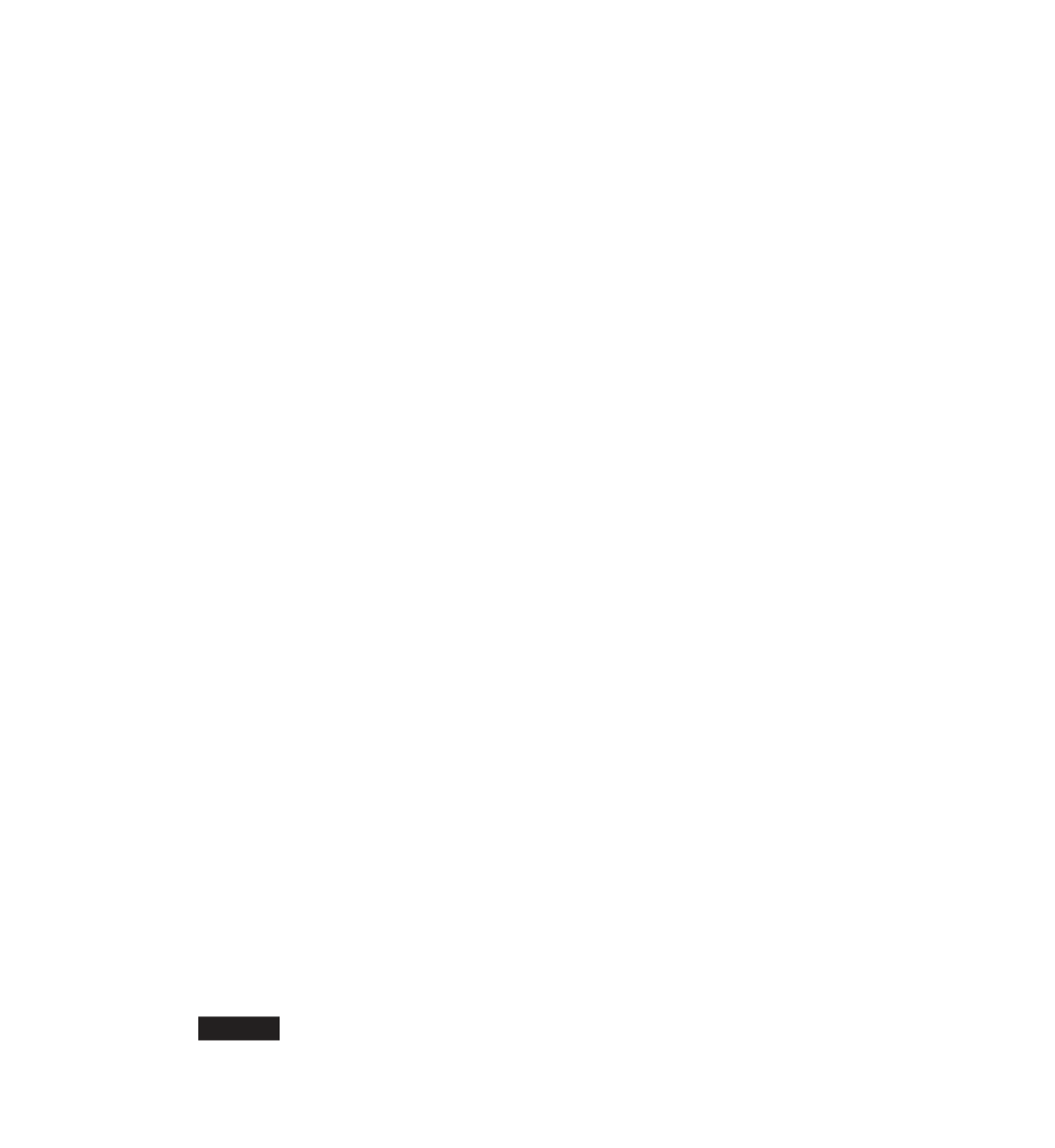



Search WWH ::

Custom Search