HTML and CSS Reference
In-Depth Information
How Emscripten Works
Under the hood, Emscripten compiles C++ into JavaScript in two main phases. First, Emscripten invokes LLVM's
Clang to compile your C and C++ source code into LLVM IR, which is a representation of a program somewhere
between source code and compiled object code. Afterwards, Emscripten translates the LLVM IR into optimized
JavaScript (see Figure
18-1
).
Figure 18-1.
The Emscripten compiler workflow
Let's walk through the steps one by one.
Clang
Clang is an open source C and C++ compiler. It translates your source code into LLVM IR, which is basically a typed,
hardware-independent assembly language (see Figure
18-2
). LLVM IR defines functions, and its typed local variables
roughly correspond to CPU registers. For example, consider a C function named
lerp
to linearly interpolate two
floating point numbers, as shown in Listing 18-1.
Figure 18-2.
The Clang stage
Listing 18-1.
lerp in C
float lerp(float a, float b, float t) {
return (1 - t) * a + t * b;
}
The same
lerp
function would be represented in LLVM IR as shown in Listing 18-2.
Listing 18-2.
lerp in LLVM IR
define internal hidden float @_lerp(float %a, float %b, float %t) nounwind readnone inlinehint ssp {
%1 = fsub float 1.000000e+00, %t
%2 = fmul float %1, %a
%3 = fmul float %t, %b
%4 = fadd float %2, %3
ret float %4
}
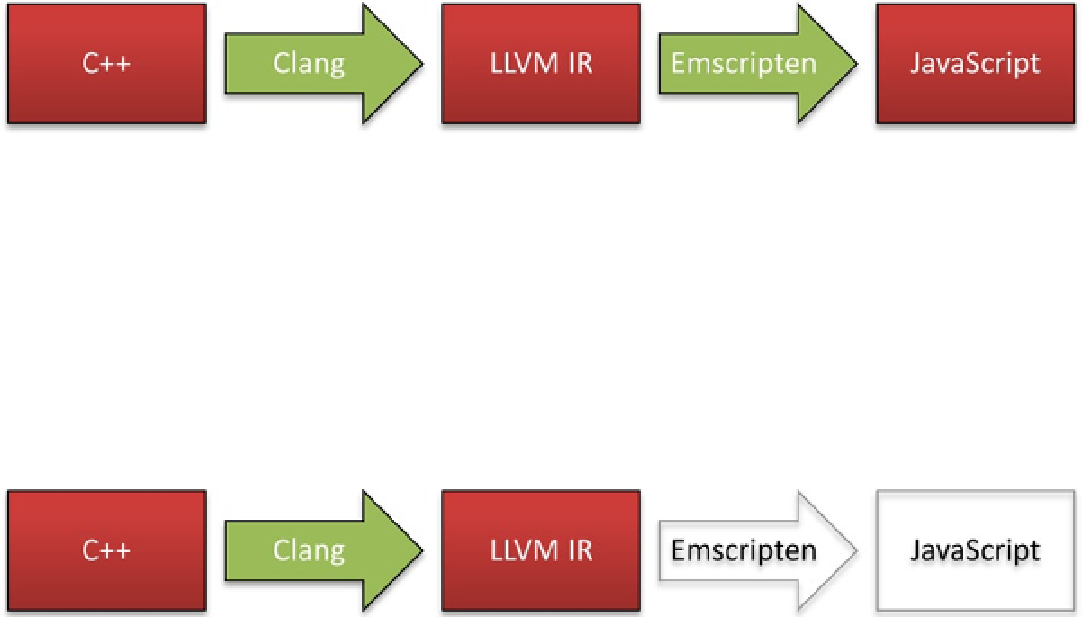