Java Reference
In-Depth Information
There is also a constructor with one additional
String
parameter for the string ini-
tially displayed in the text area. For example:
JTextArea theText =
new
JTextArea("Enter\ntext here.", 5, 20);
Note that a string value can be multiple lines because it can contain the new-line char-
acter
'\n'
.
A
JTextField
has a similar constructor with a
String
parameter, as in the following
example:
JTextField ioField =
new
JTextField("Enter numbers here.",
30);
If you look at Display 17.12, you will see that both
JTextField
and
JTextArea
are derived classes of the abstract class
JTextComponent
. Most of the methods for
JTextField
and
JTextArea
are inherited from
JTextComponent
and so
JTextField
and
JTextArea
have mostly the same methods with the same meanings except for
minor redefinitions to account for having just one line or multiple lines. Display
17.18 describes some methods in the class
JTextComponent
. All of these methods are
inherited and have the described meaning in both
JTextField
and
JTextArea.
You can set the line-wrapping policy for a
JTextArea
using the method
setLine-
Wrap
. The method takes one argument of type
boolean
. If the argument is
true
, then
at the end of a line, any additional characters for that line will appear on the following
line of the text area. If the argument is
false
, the extra characters will be on the same
line and will not be visible. For example, the following sets the line wrap policy for the
JTextArea
object named
theText
so that at the end of a line, any additional characters
for that line will appear on the following line:
theText.setLineWrap(
true
);
setLineWrap
You can specify that a
JTextField
or
JTextArea
cannot be written in by the user.
To do so, use the method
setEditable
, which is a method in both the
JTextField
and
JTextArea
classes. If
theText
names an object in either of the classes
JTextField
or
JTextArea
, then the following
theText.setEditable(
false
);
output-only
setEditable
will set
theText
so that only your GUI program can change the text in the text
component
theText
; the user cannot change the text. After this invocation of
set-
Editable
, if the user clicks the mouse in the text component named
theText
and
then types at the keyboard, the text in the text component will not change.
To reverse things and make
theText
so that the user can change the text in the text
component, use
true
in place of
false
, as follows:
theText.setEditable(
true
);
If no invocation of
setEditable
is made, then the default state allows the user to
change the text in the text component.
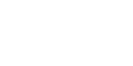
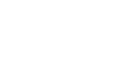


















