Java Reference
In-Depth Information
the
TreeSet<T>
class in this text, but you should be aware of their existence so you know
what to look for in the Java documentation should you need them.
Display 16.7
Methods in the Classes
ArrayList<T>
and
Vector<T>
(part 1 of 4)
The
ArrayList<T>
and
Vector<T>
classes and the
Iterator<T>
and
ListIterator<T>
inter-
faces are in the
java.util
package.
All the exception classes mentioned are unchecked exceptions, which means they are not
required to be caught in a
catch
block or declared in a
throws
clause. (If you have not yet studied
exceptions, you can consider the exceptions to be run-time error messages.)
NoSuchElementException
is in the
java.util
package, which requires an import statement if
your code mentions the
NoSuchElementException
class. All the other exception classes men-
tioned are in the package
java.lang
and so do not require any import statement.
CONSTRUCTORS
public
ArrayList(
int
initialCapacity)
Creates an empty
ArrayList<T>
with the specified initial capacity. When the
ArrayList<T>
needs to increase its capacity, the capacity doubles.
public
ArrayList( )
Creates an empty
ArrayList<T>
with an initial capacity of
10
. When the
ArrayList<T>
needs
to increase its capacity, the capacity doubles.
public
ArrayList(Collection<?
extends
T> c)
Creates a
ArrayList<T>
that contains all the elements of the collection
c
,
in the same order. In
other words, the elements have the same index in the
ArrayList<T>
created as they do in
c
.
This is not quite a
true
copy constructor because it does not preserve capacity. The capacity of
the created list will be
c.size( )
, not
c.capacity
.
The
ArrayList<T>
created is only a shallow copy of the collection argument. The
ArrayList<T>
created contains references to the elements in
c
(not references to clones of the elements in
c
).
public
Vector(
int
initialCapacity)
Creates an empty vector with the specified initial capacity. When the vector needs to increase its
capacity, the capacity doubles.
public
Vector( )
Creates an empty vector with an initial capacity of 10. When the vector needs to increase its
capacity, the capacity doubles.
public
Vector(Collection<?
extends
T> c)
Creates a vector that contains all the elements of the collection
c
, in the same order. In other
words, the elements have the same index in the vector created as they do in c
.
This is not quite a
true
copy constructor because it does not preserve capacity. The capacity of the created vector
will be
c.size( )
, not
c.capacity
.
The vector created is only a shallow copy of the collection argument. The vector created contains
references to the elements in
c
(not references to clones of the elements in
c
).
(continued)
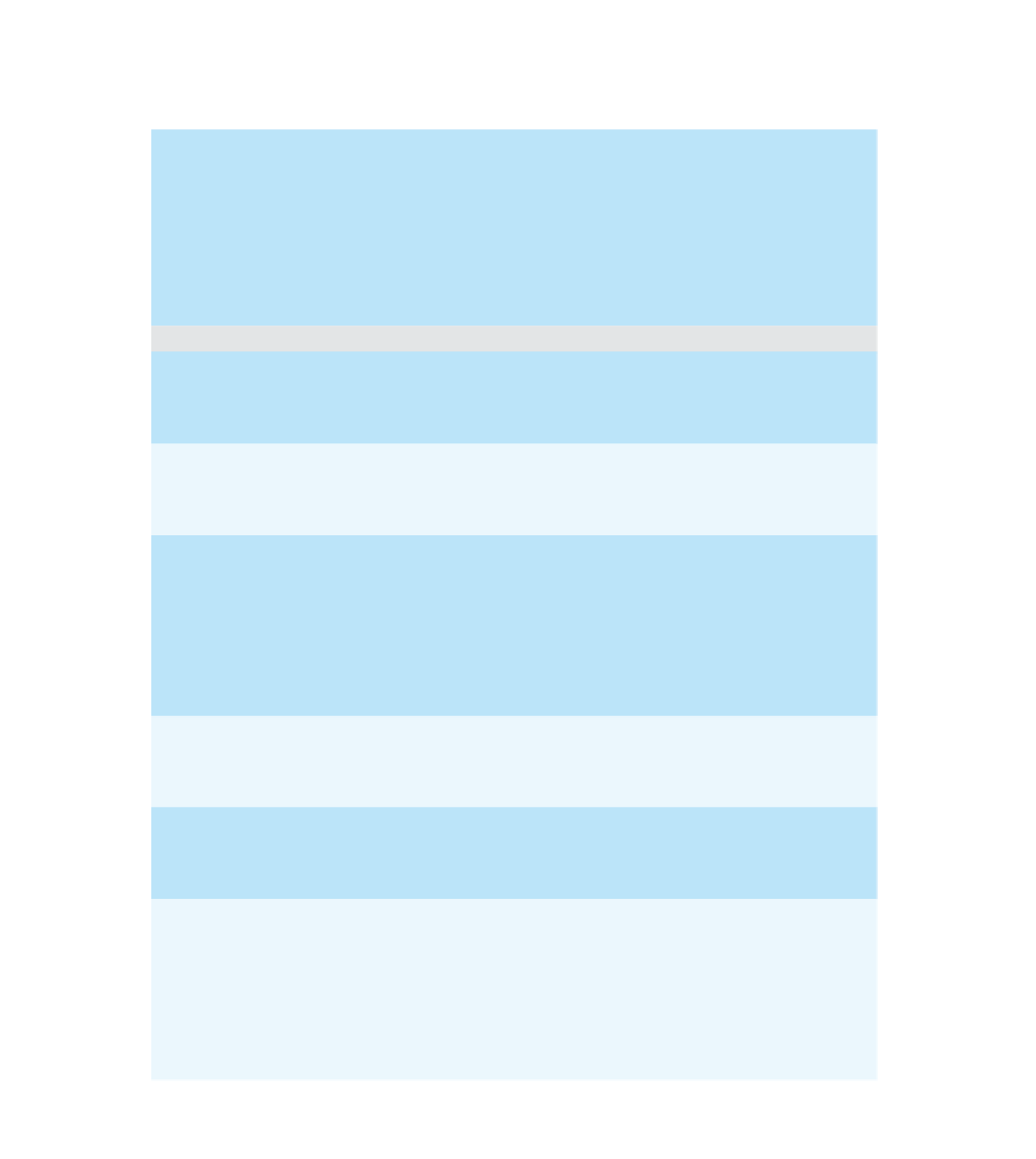

















