Java Reference
In-Depth Information
Display 15.8
A Generic Linked List Class
(part 3 of 4)
88
public
void
outputList( )
Type
T
must have well-defined
toString
methods for this to work.
89
{
90
Node<T> position = head;
91
while
(position !=
null
)
92
{
93
System.out.println(position.data);
94
position = position.link;
95
}
96
}
97
public
boolean
isEmpty( )
98
{
99
return
(head ==
null
);
100
}
101
public
void
clear( )
102
{
103
head =
null
;
104
}
105
/*
106
For two lists to be equal they must contain the same data items in
107
the same order. The equals method of T is used to compare data items.
108
*/
109
public
boolean
equals(Object otherObject)
110
{
111
if
(otherObject ==
null
)
112
return
false
;
113
else
if
(getClass( ) != otherObject.getClass( ))
114
return
false
;
115
else
116
{
117
LinkedList3<T> otherList = (LinkedList3<T>)otherObject;
118
if
(size( ) != otherList.size( ))
119
return
false
;
120
Node<T> position = head;
121
Node<T> otherPosition = otherList.head;
122
while
(position !=
null
)
123
{
124
if
(!(position.data.equals(otherPosition.data)))
125
return
false
;
126
position = position.link;
127
otherPosition = otherPosition.link;
128
}
129
return
true
;
//no mismatch was not found
130
}
131
}
132
}



















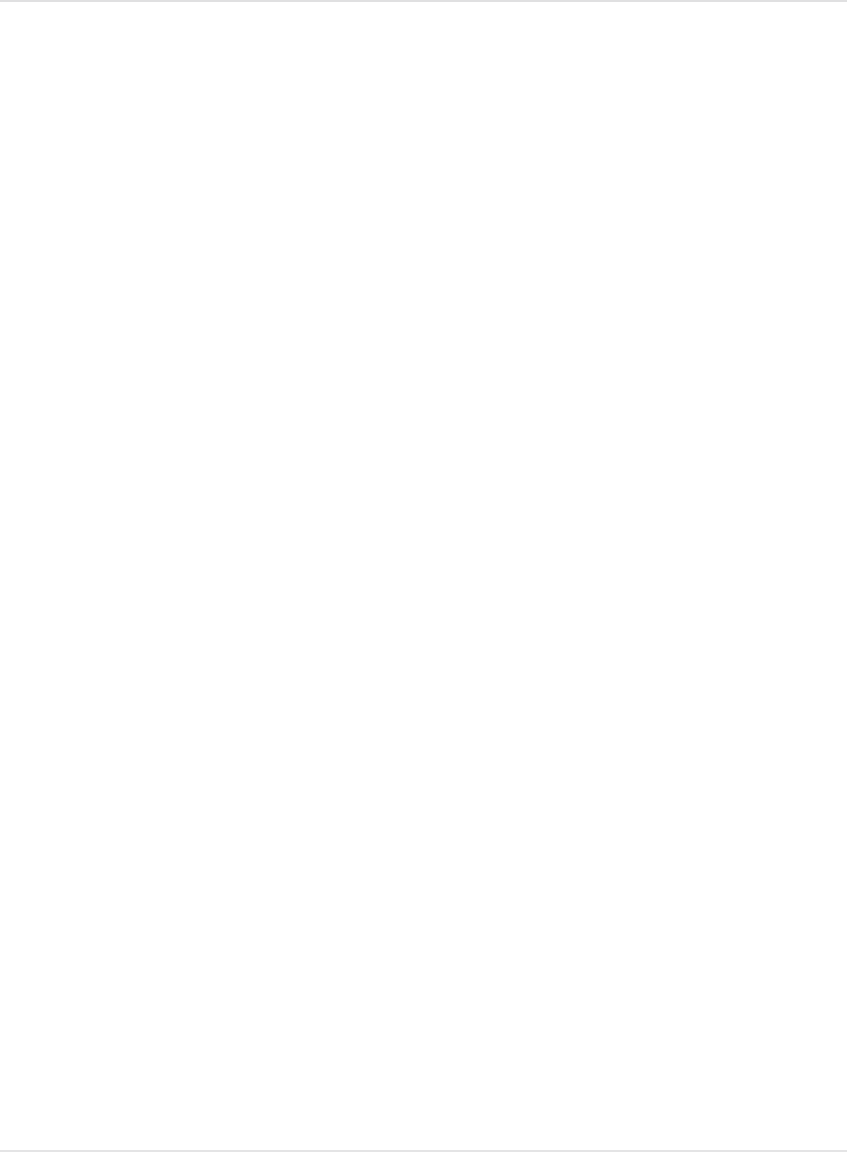