Java Reference
In-Depth Information
Flesh out the class with appropriate accessors, constructors, and mutatators.
The
uniqueItemID
's are assigned by your store and can be set from outside
the
InventoryItem
class—your code does not have to ensure that they are
unique. Next, modify the class so that it implements the
Comparable
interface.
The
compareTo()
method should compare the
uniqueItemID
's; e.g., the
InventoryItem
with item ID 5 is less than the
InventoryItem
with ID 10. Test
your class by creating an array of sample
InventoryItem
's and sort them using a
sorting method that takes as input an array of type
Comparable
.
3.
Listed below is a code skeleton for an interface called
Enumeration
and a class called
NameCollection
.
Enumeration
provides an interface to sequentially iterate through
some type of collection. In this case, the collection will be the class
NameCollection
that simply stores a collection of names using an array of strings.
interface
Enumeration
{
// Returns true if another element in the collection exists
public boolean
hasNext();
// Returns the next element in the collection as an Object
public
Object getNext();
}
/**
* NameCollection implements a collection of names using
* a simple array.
*/
class
NameCollection
{
String[] names;
/**
* The list of names is initialized from outside
* and passed in as an array of strings
*/
NameCollection(String[] names)
{
this.names = names;
}
/**
* getEnumeration should return an instance of a class that implements
* the Enumeration interface where hasNext() and getNext()
* correspond to data stored within the names array.
*/
Enumeration getEnumeration ()
{
// Complete code here using an inner class
}
}
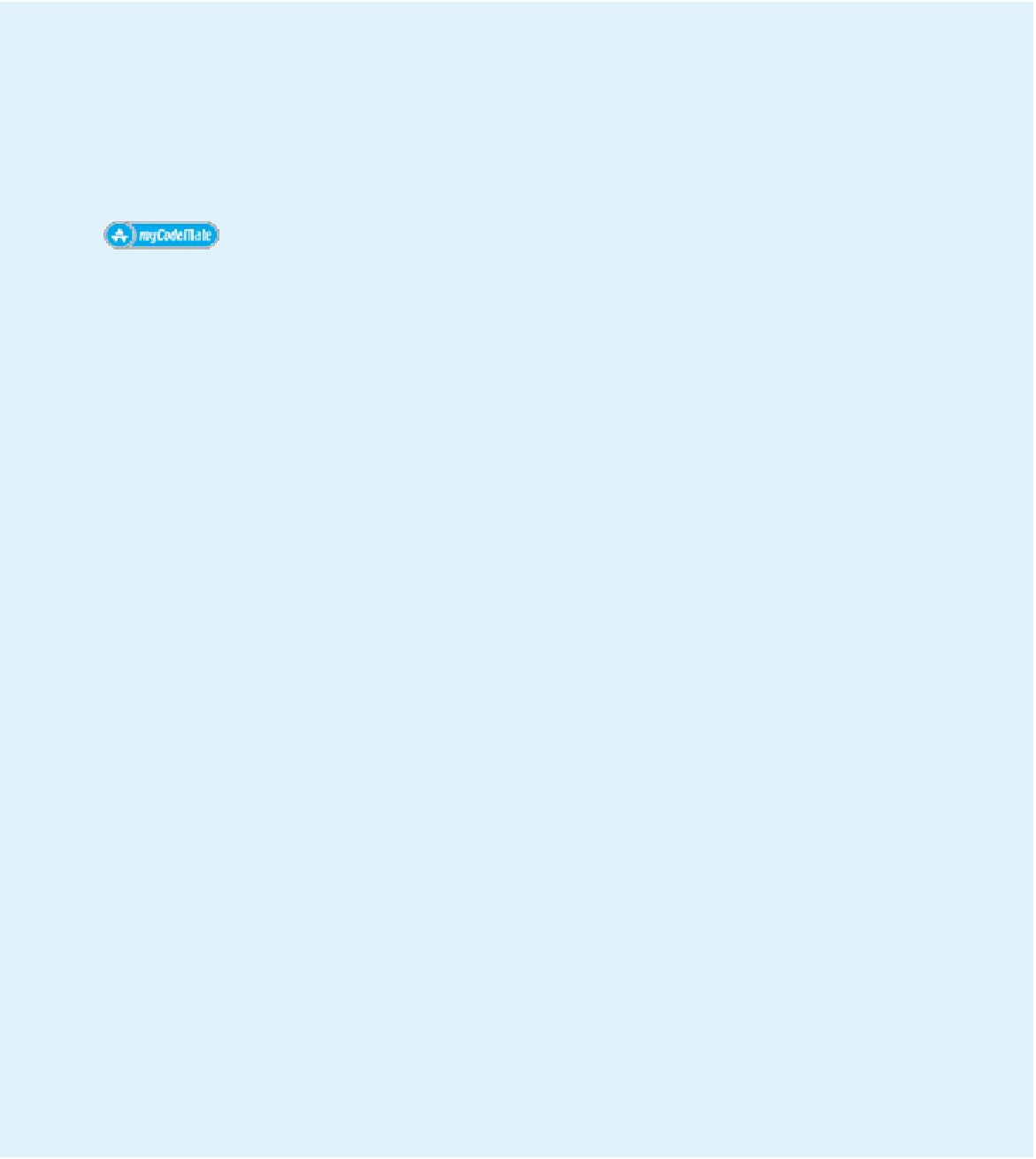

















