Java Reference
In-Depth Information
Write a program that inputs the amount of money to deposit, an interest rate per
year, and the number of years the money will accrue compound interest. Write a
recursive function that calculates the amount of money that will be in the savings
account using the input information.
To verify your function, the amount should be equal to
P(1+i)
n
, where
P
is the
amount initially saved,
i
is the interest rate per year, and
n
is the number of
years.
2.
There are
n
people in a room, where
n
is an integer greater than or equal to 1.
Each person shakes hands once with every other person. What is the total num-
ber,
h(n)
of handshakes? Write a recursive function to solve this problem. To get
you started, if there are only one or two people in the room, then:
handshake(1) = 0
handshake(2) = 1
If a third person enters the room, she must shake hands with each of the two people
already there. This is two handshakes in addition to the number of handshakes that
would be made in a room of two people, or a total of three handshakes.
If a fourth person enters the room, she must shake hands with each of the three
people already present. This is three handshakes in addition to the number of
handshakes that would be made in a room of three people, or six handshakes.
If you can generalize this to
n
handshakes then it should help you write the
recursive solution.
3.
Consider a frame of bowling pins shown below, where each
*
represents a pin:
*
* *
* * *
* * * *
* * * * *
There are five rows and a total of fifteen pins.
If we had only the top four rows then there would be a total of 10 pins.
If we had only the top three rows then there would be a total of 6 pins.
If we had only the top two rows then there would be a total of 3 pins.
If we had only the top row then there would be a total of 1 pin.
Write a recursive function that takes as input the number of rows
n
and outputs
the total number of pins that would exist in a pyramid with
n
rows. Your
program should allow for values of
n
that are larger than 5.
4.
The game of “Jump It” consists of a board with
n
positive integers in a row
except for the first column, which always contains zero. These numbers repre-
sent the cost to enter each column. Here is a sample game board where
n
is 6:
0
3
80
6
57
10
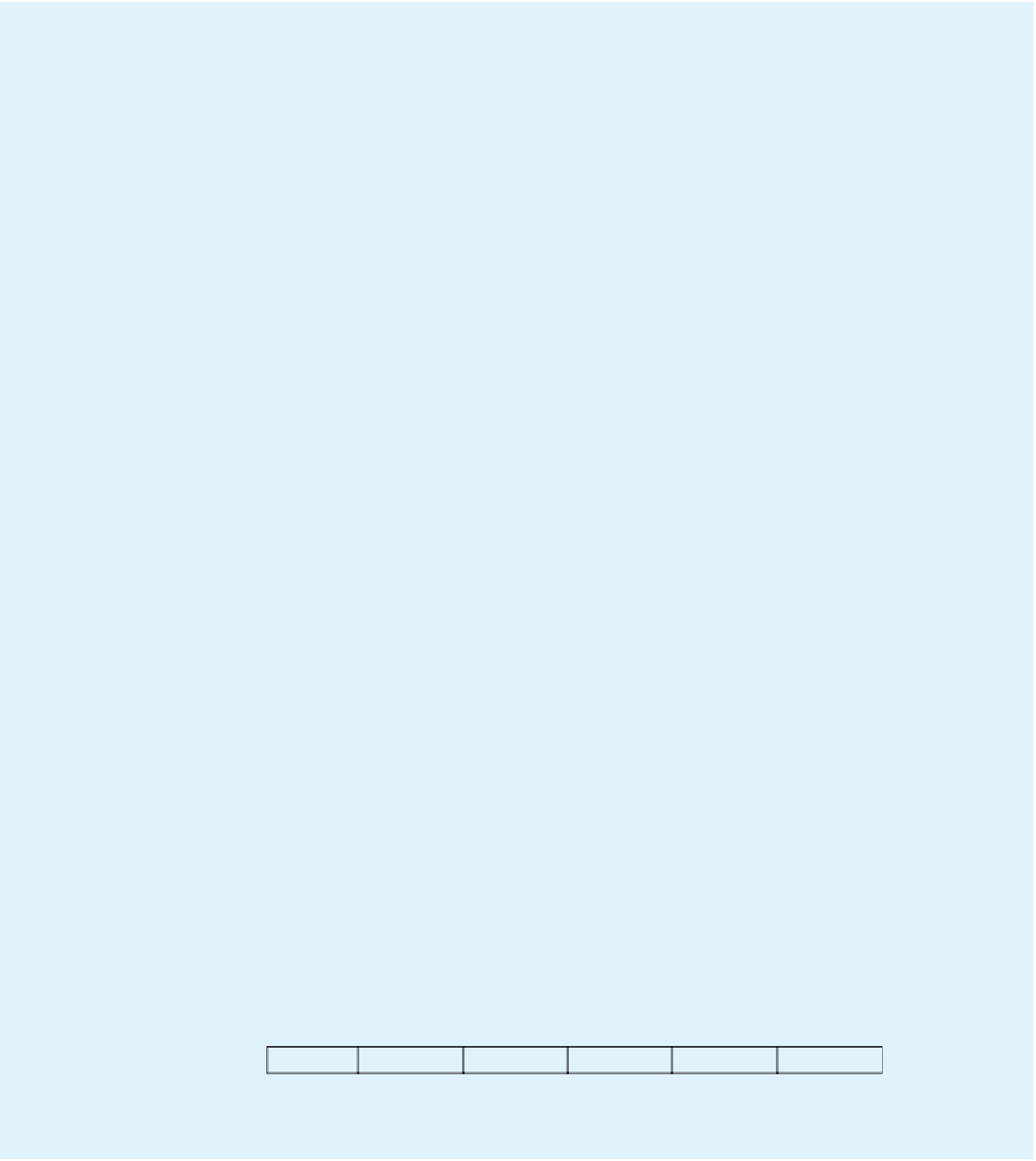
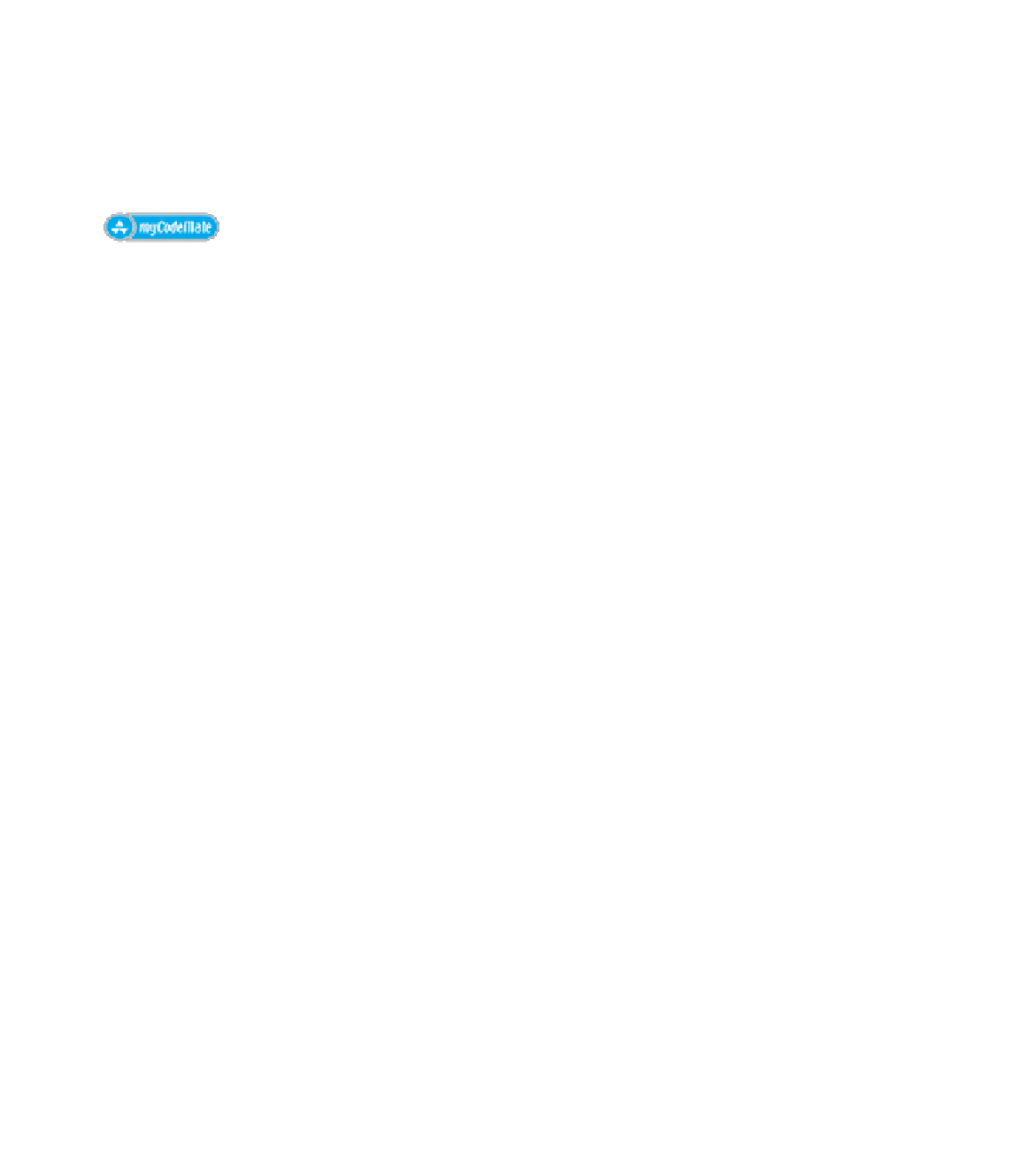

















