Java Reference
In-Depth Information
Display 10.22
Random Access to a File
(part 1 of 2)
1
import
java.io.RandomAccessFile;
2
import
java.io.IOException;
3
import
java.io.FileNotFoundException;
4
public class
RandomAccessDemo
5{
6
public static void
main(String[] args)
7 {
8
try
9
{
10
RandomAccessFile ioStream =
11
new
RandomAccessFile("bytedata", "rw");
12
System.out.println("Writing 3 bytes to the file bytedata.");
13
ioStream.writeByte(1);
14
ioStream.writeByte(2);
15
ioStream.writeByte(3);
16
System.out.println("The length of the file is now = "
17
+ ioStream.length());
18
System.out.println("The file pointer location is "
19
+ ioStream.getFilePointer());
20
System.out.println("Moving the file pointer to location 1.");
21
ioStream.seek(1);
22
byte oneByte = ioStream.readByte();
23
System.out.println("The value at location 1 is " + oneByte);
24
oneByte = ioStream.readByte();
25
System.out.println("The value at the next location is "
26
+ oneByte);
27
System.out.println("Now we move the file pointer back to");
28
System.out.println("location 1, and change the byte.");
29
ioStream.seek(1);
30
ioStream.writeByte(9);
31
ioStream.seek(1);
32
oneByte = ioStream.readByte();
33
System.out.println("The value at location 1 is now " + oneByte);
34
System.out.println("Now we go to the end of the file");
35
System.out.println("and write a double.");
36
ioStream.seek(ioStream.length());
37
ioStream.writeDouble(41.99);
38
System.out.println("The length of the file is now = "
39
+ ioStream.length());




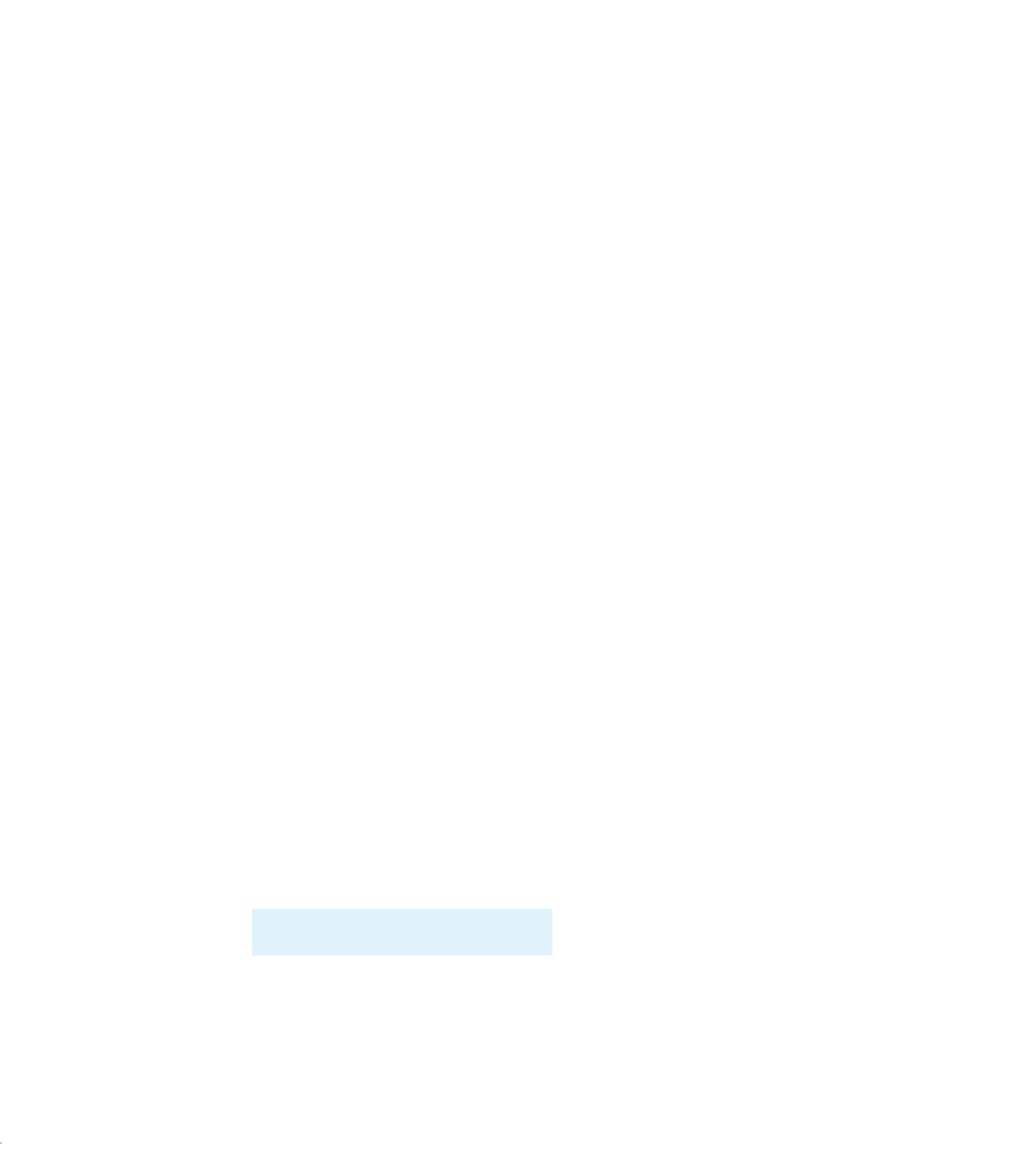

















