Java Reference
In-Depth Information
IOException
When dealing with file I/O, there are many situations in which your code might throw an
exception of some class, such as
FileNotFoundException
. Many of these various excep-
tion classes are descended classes of the class
IOException
. The class
IOException
is the
root class for various exception classes having to do with input and output.
A
FileNotFoundException
is thrown if it is impossible to create the file—for example,
because the file name is already used for a directory (folder) name.
A
FileNotFoundException
is a kind of
IOException
, so a
catch
block for an
IOException
would also work and would look more sensible. However, it is best to
catch the most specific exception that you can, since that can give more information.
As illustrated in Display 10.1, the method
println
of the class
PrintWriter
works the same for writing to a text file as the method
System.out.println
works
for writing to the screen. The class
PrintWriter
also has the method
print
and
printf
, which behaves just like
System.out.print
and
System.out.printf
except
that the output goes to a text file. Display 10.2 describes some of the methods in the
class
PrintWriter
.
println
print
printf
Display 10.2
Some Methods of the Class
PrintWriter
(part 1 of 2)
PrintWriter
and
FileOutputStream
are in the
java.io
package.
public
PrintWriter(OutputStream streamObject)
This is the only constructor you are likely to need. There is no constructor that accepts a file name as
an argument. If you want to create a stream using a file name, you use
new
PrintWriter(
new
FileOutputStream(
File_Name
))
When the constructor is used in this way, a blank file is created. If there already was a file named
File_Name
, then the old contents of the file are lost. If you want instead to append new text to
the end of the old file contents, use
new
PrintWriter(
new
FileOutputStream(
File_Name,
true
))
(For an explanation of the argument
true
, read the subsection “Appending to a Text File.“)
When used in either of these ways, the
FileOutputStream
constructor, and so the
PrintWriter
constructor invocation, can throw a
FileNotFoundException
, which is a kind of
IOException
.
If you want to create a stream using an object of the class
File
, you can use a
File
object in place
of the
File_Name
. (The
File
class will be covered in Section 10.3. We discuss it here so that you will
have a more complete reference in this display, but you can ignore the reference to the class
File
until after you've read that section.)
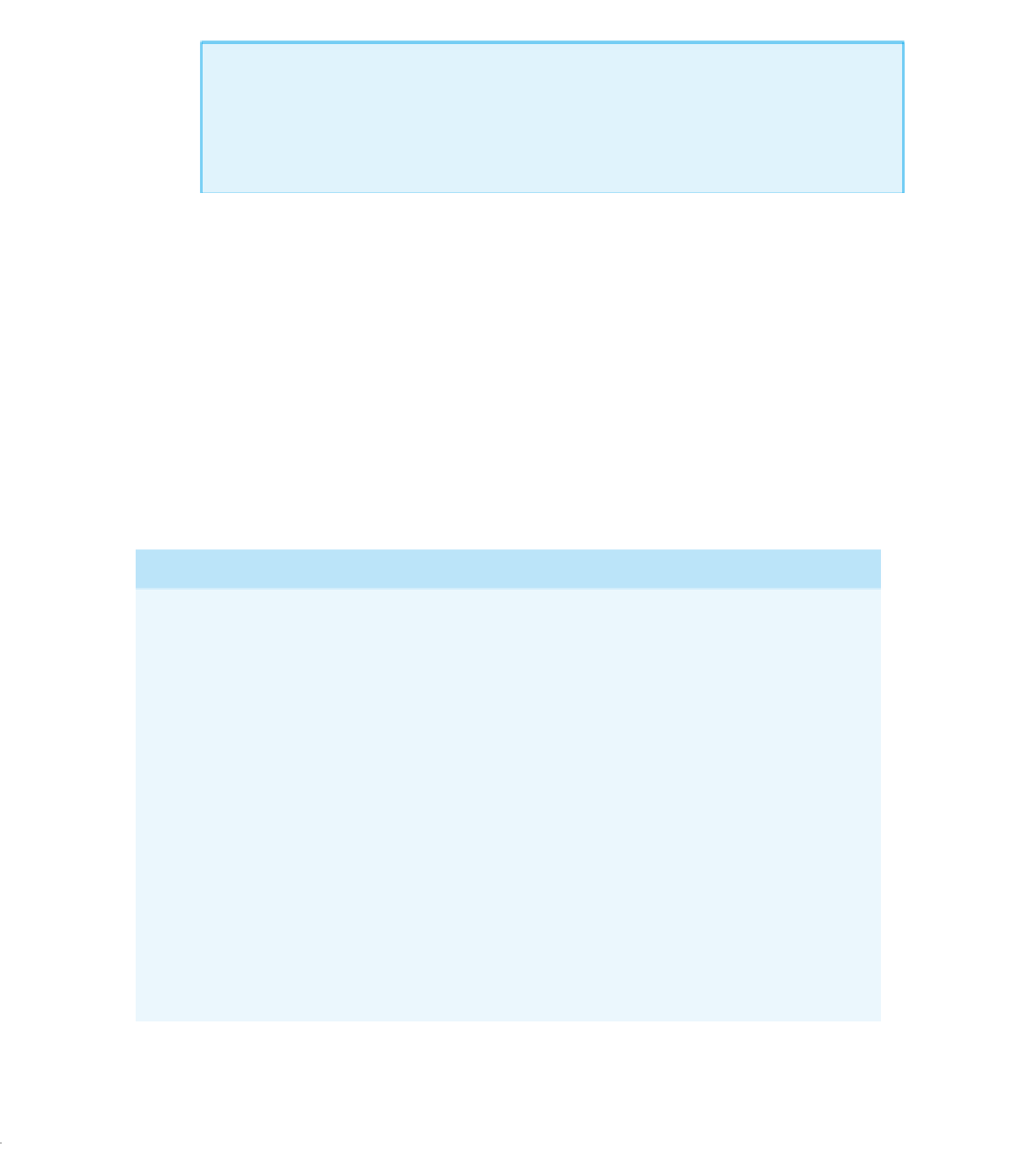

















