Java Reference
In-Depth Information
The Class
Object
In Java, every class is a descendent of the class
Object
. So, every object of every class is of
type
Object
, as well as being of the type of its class.
The class
Object
is in the package
java.lang
, which is always imported automati-
cally. So, you do not need any
import
statement to make the class
Object
available to
your code.
The class
Object
does have some methods that every Java class inherits. For exam-
ple, every object inherits the methods
equals
and
toString
from some ancestor class,
which either is the class
Object
or a class that itself inherited the methods ultimately
from the class
Object
. However, the inherited methods
equals
and
toString
will not
work correctly for (almost) any class you define. You need to override the inherited
method definitions with new, more appropriate definitions.
It is important to include definitions of the methods
toString
and
equals
in the
classes you define, since some Java library classes assume your class has such methods.
There are no subtleties involved in defining (actually redefining or overriding) the
method
toString
. We have seen good examples of the method
toString
in many of
our class definitions. The definition of the overridden method
equals
does have some
subtleties and we will discuss them in the next subsection.
Another method inherited from the class
Object
is the method
clone
, which is
intended to return a copy of the calling object. We discuss the method
clone
in Chap-
ters 8 and 13.
toString
equals
clone
The Right Way to Define
equals
Earlier we said that the class
Object
has an
equals
method, and that when you define
a class with an
equals
method you should override the definition of the method
equals
given in the class
Object
. However, we did not, strictly speaking, follow our
own advice. The heading for the method
equals
in our definition of the class
Employee
(Display 7.2) is as follows:
public
boolean
equals(Employee otherEmployee)
On the other hand, the heading for the method
equals
in the class
Object
is as follows:
public boolean
equals(Object otherObject)
The two
equals
methods have different parameter types, so we have not overridden
the definition of
equals
. We have merely overloaded the method
equals
. The class
Employee
has both of these methods named
equals
.

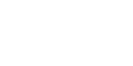


















