Java Reference
In-Depth Information
Display 7.5
The Derived Class
SalariedEmployee
(part 1 of 2)
1
/**
2
Class Invariant: All objects have a name string, hire date,
3
and nonnegative salary. A name string of "No name" indicates
4
no real name specified yet. A hire date of January 1, 1000 indicates
5
no real hire date specified yet.
6
*/
7
public
class
SalariedEmployee
extends
Employee
8{
9
private
double
salary;
//annual
It will take the rest of Section 7.1 to
fully explain this class definition.
10
public
SalariedEmployee()
11
{
12
super
();
13
salary = 0;
If this line is omitted, Java will still invoke
the no-argument constructor for the
base class.
14
}
15
/**
16
Precondition: Neither theName nor theDate are null;
17
theSalary is nonnegative.
18
*/
19
public
SalariedEmployee(String theName, Date theDate,
double
theSalary)
20
{
21
super
(theName, theDate);
22
if
(theSalary >= 0)
23
salary = theSalary;
24
else
25
{
26
System.out.println("Fatal Error: Negative salary.");
27
System.exit(0);
28
}
29
}
30
public
SalariedEmployee(SalariedEmployee originalObject )
31
{
An object of the class
SalariedEmployee
is also an
object of the class
Employee
.
32
super
(originalObject);
33
salary = originalObject.salary;
34
}
35
public
double
getSalary()
36
{
37
return
salary;
38
}
(continued)





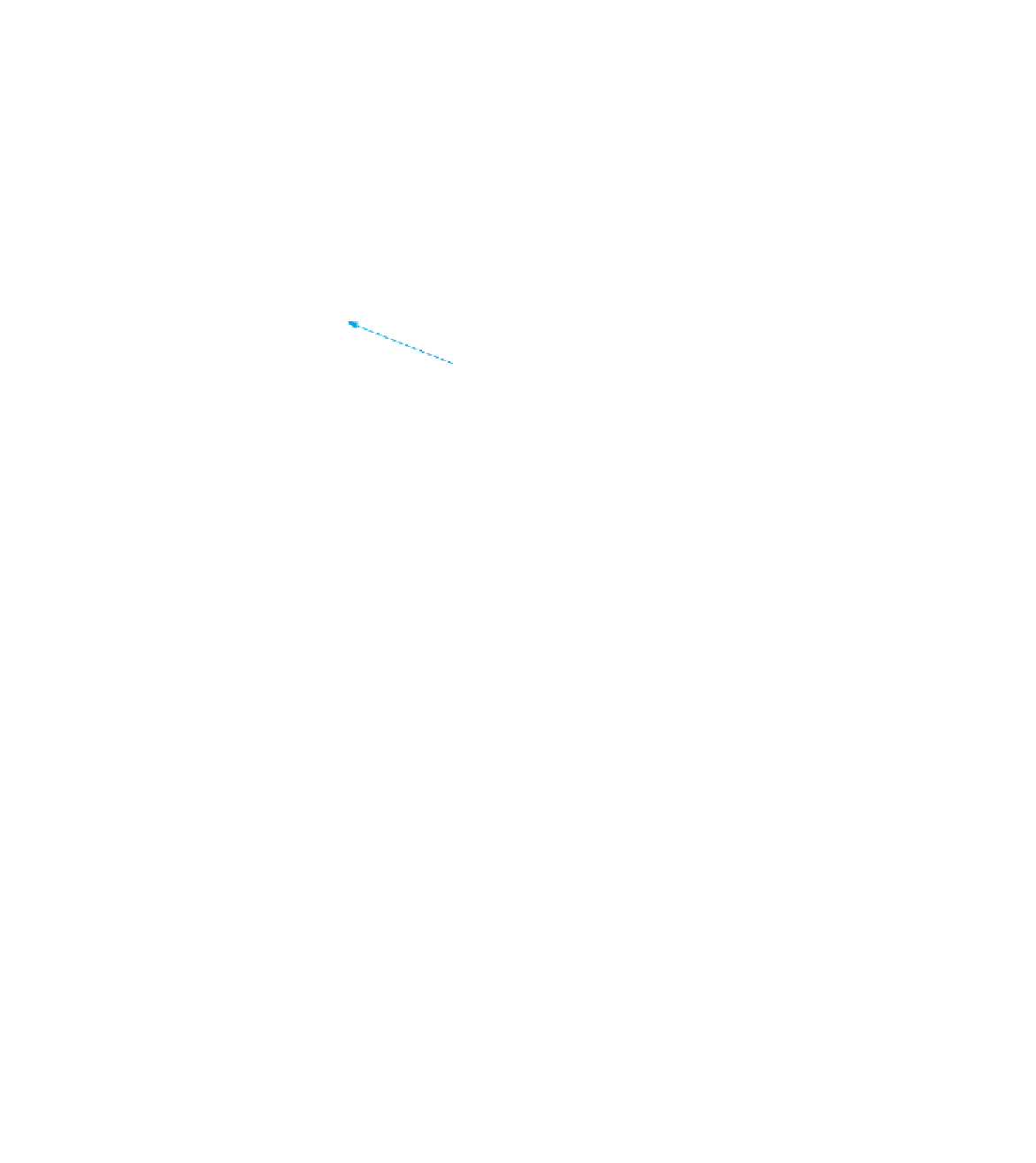



















