Java Reference
In-Depth Information
indices for the elements, as there are with an array. This new kind of
for
loop is called
a
for-each loop
or
enhanced for loop
. We will discuss these for-each loops in detail
when we cover collections (Chapter 15). However, these new for-each loops can be
used with arrays as well as with objects of these collection classes. In this subsection,
we tell you how to use for-each loops with arrays in case you want to get started using
them. However, we do not use the for-each loop in this topic until we discuss collec-
tion classes in Chapter 15.
The following code ends with a for-each loop that is equivalent to the regular
for
loop that we gave at the start of this subsection:
for-each loop
double
[] a =
new double
[10];
<
Some code to fill the array
a>
for
(
double
element : a)
System.out.println(element);
You can read the line beginning with
for
as “for each
element
in
a
, do the follow-
ing.” Note that the variable,
element
, has the same type as the elements in the array.
The variable, like
element
, must be declared in the for-each loop as we have done. If
you attempt to declare
element
before the for-each loop, you will get a compiler
error message.
The general syntax for a for-each loop statement used with an array is
for
(
Array_Base_Type
Variable
:
Array_Name
)
Statement
Be sure to notice that you use a colon (not a semicolon) after the
Variable
. You may
use any legal variable name for the
Variable
; you do not have to use
element
.
Although it is not required, the
Statement
typically contains the
Variable
. When the
for-each loop is executed, the
Statement
is executed once for each element of the
array. More specifically, for each
element
of the array, the
Variable
is set to the array
element and then the
Statement
is executed.
The for-each loop can make your code a lot cleaner and a lot less error prone. If
you are not using the indexed variable in a
for
loop for anything other than as a
way to cycle through all the array elements, then a for-each loop is preferable. For
example,
for
(
double
element : a)
element = 0.0;
is preferable to
for
(
int
i = 0; i < a.length; i++)
a[i]= 0.0;
The two loops do the same thing, but the second one mentions an index
i
that is not
used for anything other than enumerating the array elements. Also, the syntax for the
for-each loop is simpler than that of the regular
for
loop.
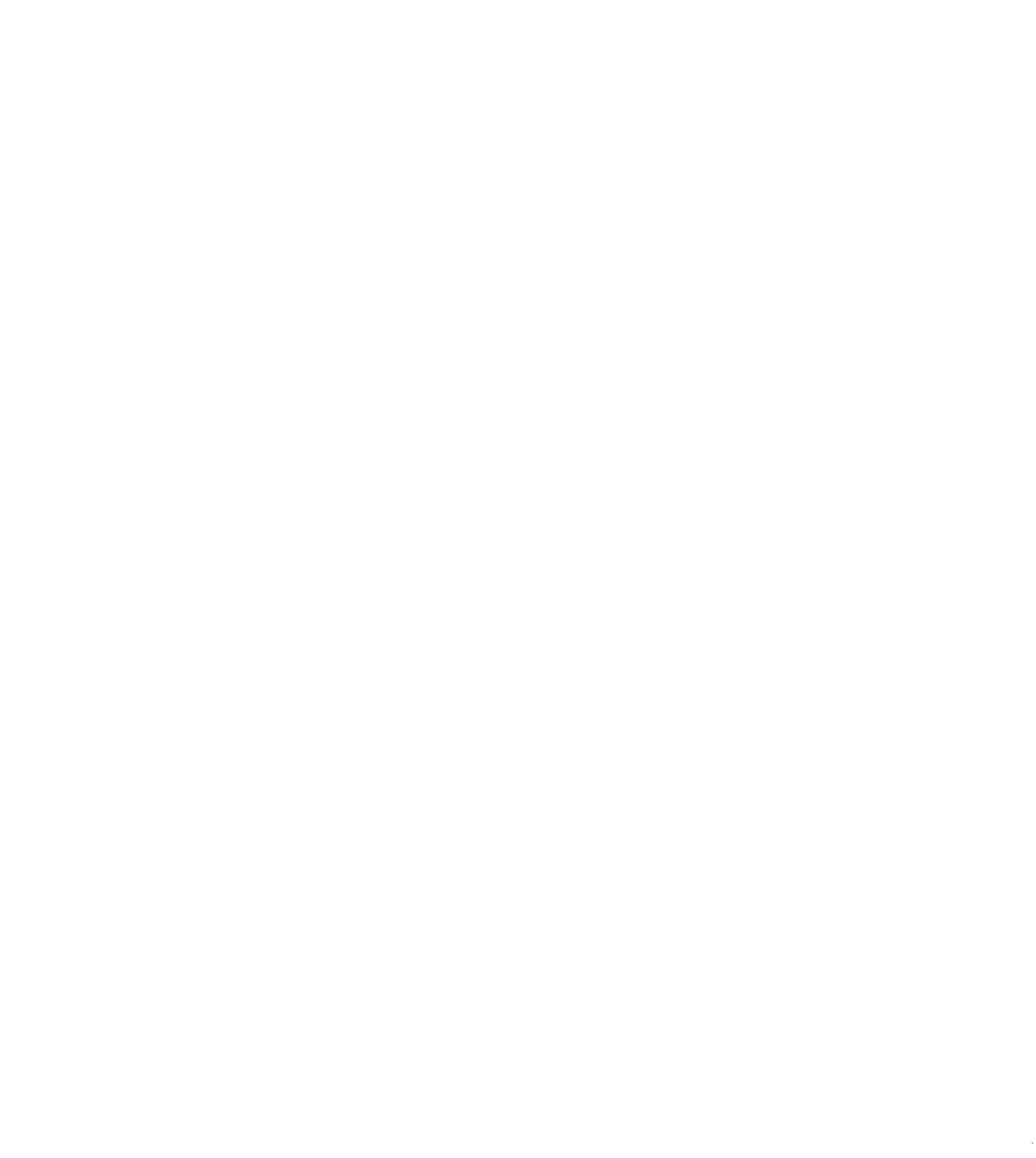

















