Java Reference
In-Depth Information
Self-Test Exercises
(continued)
Which of the following are acceptable method calls?
int[]
a = {4, 5, 6};
int
number = 2;
SomeClass.doSomething(number);
SomeClass.doSomething(a[2]);
SomeClass.doSomething(a[3]);
SomeClass.doSomething(a[number]);
SomeClass.doSomething(a);
8. Write a method definition for a static
void
method called
oneMore
, which has a
formal parameter for an array of integers and increases the value of each array ele-
ment by one. (The definition will go in some class, but you need only give the
method definition.)
9. Write a method named
outOfOrder
that takes as a parameter an array of
double
and returns a value of type
int
. This method will test the array for being out of
order, meaning that the array violates the condition:
a[0] <= a[1] <= a[2] <= ...
The method returns
−
1
if the elements are not out of order; otherwise, it returns
the index of the first element of the array that is out of order. For example,
consider the declaration
double
[] a
= {1.2, 2.1, 3.3, 2.5, 4.5,
7.9, 5.4, 8.7, 9.9, 1.0};
In the array above,
a[2]
and
a[3]
are the first pair out of order, and
a[3]
is the
first element out of order, so the method returns
3
. If the array were sorted, the
method would return
−
1
.
10. What is wrong with the following method definition? It will compile but does not
work as you might hope.
public static void
doubleSize(
int
[] a)
{
a =
new int
[a.length * 2];
}
Array Parameters and Array Arguments
An argument to a method may be an entire array. Array arguments are like objects of a class,
in that the method can change the data in an array argument; that is, a method can change
the values stored in the indexed variables of an array argument. A method with an array
parameter is defined and invoked as illustrated by the following examples. Note that the array
parameter specifies the base type of the array, but not the length of the array.

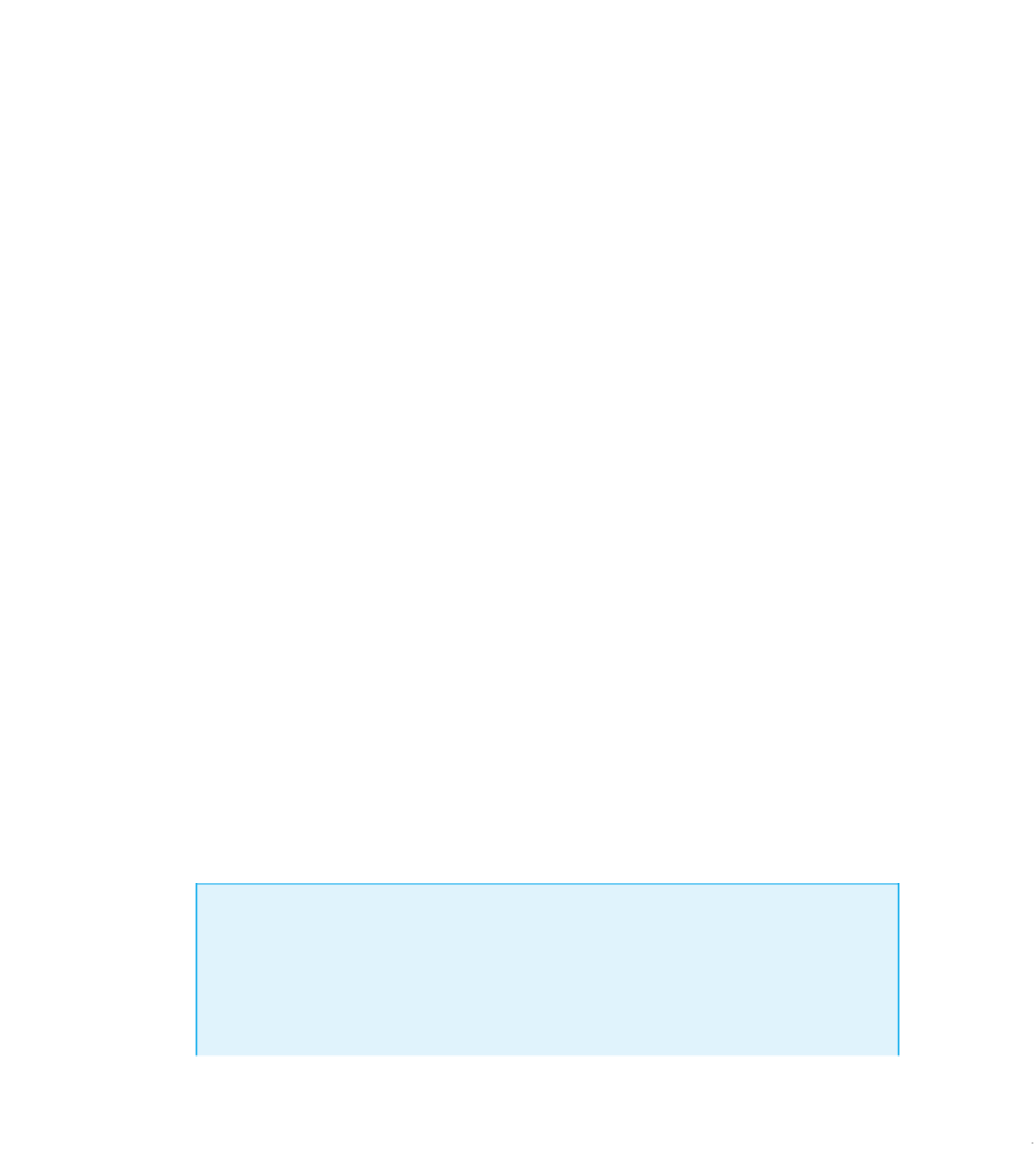

















