Java Reference
In-Depth Information
Copy Constructors
A
copy constructor
is a constructor with a single argument of the same type as the class.
The copy constructor should create an object that is a separate, independent object but
with the instance variables set so that it is an exact copy of the argument object.
For example, Display 5.20 reproduces the copy constructor for the class
Date
defined in Display 4.11. The copy constructor, or any other constructor, creates a new
object of the class
Date
. That part happens automatically and is not shown in the code
for the copy constructor. The code for the copy constructor then goes on to set the
instance variables to the values equal to those of its one parameter,
aDate
. But the new
date created is a separate object even though it represents the same date. Consider the
following code:
copy
constructor
Date date1 =
new
Date("January", 1, 2006);
Date date2 =
new
Date(date1);
After this code is executed, both
date1
and
date2
represent the date January 1, 2006,
but they are two different objects. So, if we change one of these objects, it will not
change the other. For example, consider
date2.setDate("July", 4, 1776);
System.out.println(date1);
The output produced is
January 1, 2006
When we changed
date2
, we did not change
date1
. This may not be a difficult or even
subtle point, but it is critically important to much of what we discuss in this section of
the chapter. (See Self-Test Exercise 39 in this chapter to see the copy constructor con-
trasted with the assignment operator.)
Now let's consider the copy constructor for the class
Person
(Display 5.19), which
is a bit more complicated. It is reproduced in what follows:
public
Person(Person original)
{
if
(original ==
null
)
{
System.out.println("Fatal error.");
System.exit(0);
}
name = original.name;
born =
new
Date(original.born);
if
(original.died ==
null
)
died =
null
;
else
died =
new
Date(original.died);
}
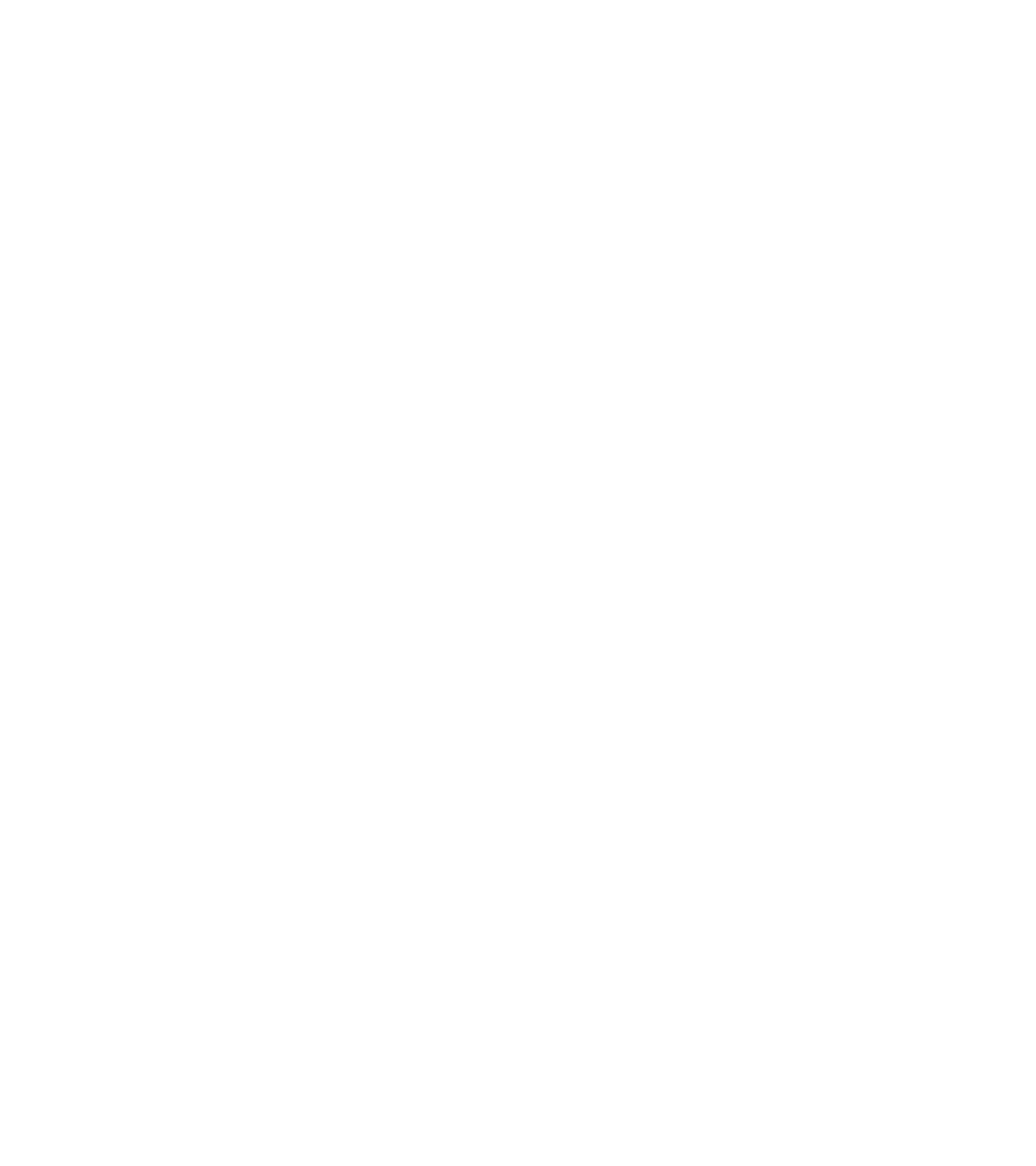











