Java Reference
In-Depth Information
Here are additional examples to illustrate the differences between the prefix form of
++
(or
——
) and the postfix form of
++
(or
——
). Consider the following code:
int
i
=
10;
Same effect as
int
newNum =
10
* i;
i = i + 1;
int
newNum =
10
* i++;
System.out.print(
"i is "
+ i
+
", newNum is "
+ newNum);
i is 11, newNum is 100
In this case,
i
is incremented by
1
, then the
old
value of
i
is used in the multiplication. So
newNum
becomes
100
. If
i++
is replaced by
++i
as follows,
int
i
=
10;
Same effect as
int
newNum =
10
* (++i);
i = i + 1;
int
newNum =
10
* i;
System.out.print(
"i is "
+ i
+
", newNum is "
+ newNum);
i is 11, newNum is 110
i
is incremented by
1
, and the new value of
i
is used in the multiplication. Thus
newNum
becomes
110
.
Here is another example:
double
x =
1.0
;
double
y =
5.0
;
double
z = x-- + (++y);
After all three lines are executed,
y
becomes
6.0
,
z
becomes
7.0
, and
x
becomes
0.0
.
Tip
Using increment and decrement operators makes expressions short, but it also
makes them complex and difficult to read. Avoid using these operators in expres-
sions that modify multiple variables or the same variable multiple times, such as this
one:
int k = ++i + i
.
2.18
✓
✓
Which of these statements are true?
Check
Point
a. Any expression can be used as a statement.
b. The expression
x++
can be used as a statement.
c. The statement
x = x + 5
is also an expression.
d. The statement
x = y = x = 0
is illegal.
2.19
Assume that
int a = 1
and
double d = 1.0
, and that each expression is inde-
pendent. What are the results of the following expressions?
a =
46
/
9
;
a =
46
%
9
+
4
*
4
-
2
;
a =
45
+
43
%
5
* (
23
*
3
%
2
);
a %=
3
/ a +
3
;
d =
4
+ d * d +
4
;
d +=
1.5
*
3
+ (++a);
d -=
1.5
*
3
+ a++;
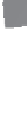
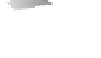


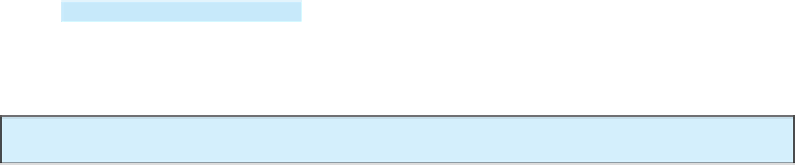
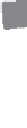







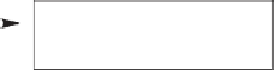
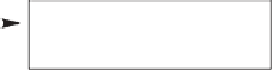





