Java Reference
In-Depth Information
The tic-tac-toe board consists of nine cells, created using
new Cell[3][3]
. To determine
which player's turn it is, you can introduce a variable named
whoseTurn
of the
char
type.
whoseTurn
is initially
'X'
, then changes to
'O'
, and subsequently changes between
'X'
and
'O'
whenever a new cell is occupied. When the game is over, set
whoseTurn
to
' '
.
How do you know whether the game is over, whether there is a winner, and who the win-
ner, if any, is? You can define a method named
isWon(char token)
to check whether a
specified token has won and a method named
isFull()
to check whether all the cells are
occupied.
Clearly, two classes emerge from the foregoing analysis. One is the
Cell
class, which han-
dles operations for a single cell; the other is the
TicTacToe
class, which plays the whole
game and deals with all the cells. The relationship between these two classes is shown in
Figure 18.12.
javax.swing.JApplet
Cell
9
1
TicTacToe
-whoseTurn: char
Indicates which player has the turn, initially X.
-cell: Cell[][]
-jlblStatus: JLabel
A 3
3, two-dimensional array for cells.
A label to display game status.
+TicTacToe()
+isFull(): boolean
Constructs the TicTacToe user interface.
Returns true if all cells are filled.
+isWon(token: char): boolean
Returns true if a player with the specified token has won.
F
IGURE
18.12
The
TicTacToe
class contains nine cells.
Since the
Cell
class is only to support the
TicTacToe
class, it can be defined as an inner
class in
TicTacToe
. The complete program is given in Listing 18.10.
L
ISTING
18.10
TicTacToe.java
1
import
java.awt.*;
2
import
java.awt.event.*;
3
import
javax.swing.*;
4
import
javax.swing.border.LineBorder;
5
6
public class
TicTacToe
extends
JApplet
{
main class
TicTacToe
7
// Indicate which player has a turn; initially it is the X player
8
private char
whoseTurn =
'X'
;
9
10
// Create and initialize cells
11
private
Cell[][] cells =
new
Cell[
3
][
3
];
12
13
// Create and initialize a status label
14
private
JLabel jlblStatus =
new
JLabel(
"X's turn to play"
);
15
16
/** Initialize UI */
17
public
TicTacToe()
{
18
// Panel p to hold cells

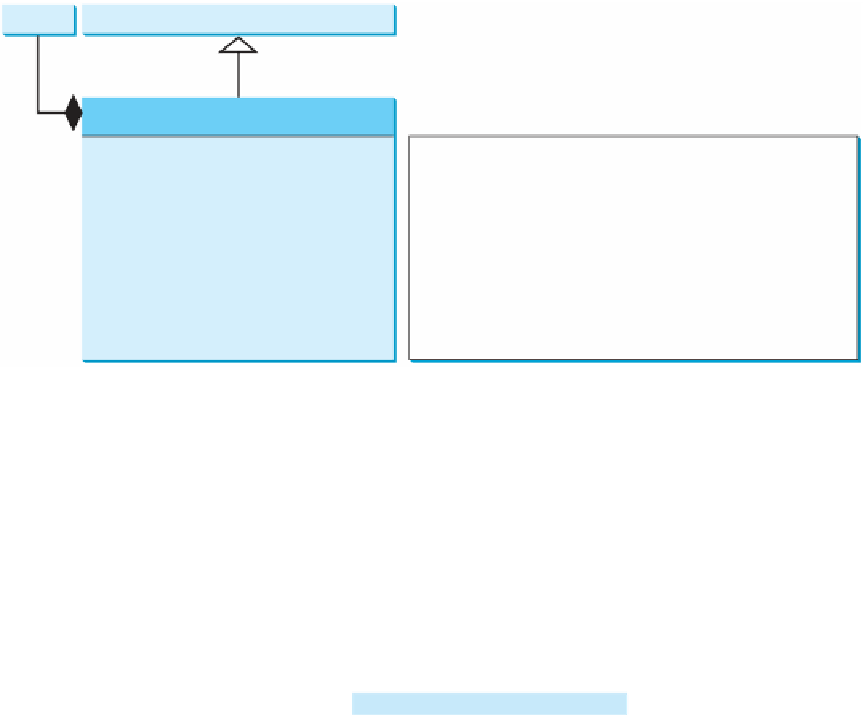