Java Reference
In-Depth Information
More details on objects will be introduced in Chapter 8. For the time being, simply accept
that this is how to obtain input from the console.
Listing 2.3 gives an example of reading multiple input from the keyboard. The program
reads three numbers and displays their average.
L
ISTING
2.3
ComputeAverage.java
1
import
java.util.Scanner;
// Scanner is in the java.util package
import class
2
3
public class
ComputeAverage {
4
public static void
main(String[] args) {
5
// Create a Scanner object
6
7
8
// Prompt the user to enter three numbers
9 System.out.print(
"Enter three numbers: "
);
10
Scanner input =
new
Scanner(System.in);
create a
Scanner
double
number1 =
input.nextDouble();
read a
double
11
double
number2 =
input.nextDouble();
12
double
number3 =
input.nextDouble();
13
14
// Compute average
15
double
average = (number1 + number2 + number3) /
3
;
16
17
// Display results
18 System.out.println(
"The average of "
+ number1 +
" "
+ number2
19 +
" "
+ number3 +
" is "
+ average);
20 }
21 }
Enter three numbers:
The average of 1.0 2.0 3.0 is 2.0
1 2 3
enter input in one line
10.5
Enter three numbers:
11
11.5
enter input in multiple lines
The average of 10.5 11.0 11.5 is 11.0
The code for importing the
Scanner
class (line 1) and creating a
Scanner
object (line 6)
are the same as in the preceding example as well as in all new programs you will write for
reading input from the keyboard.
Line 9 prompts the user to enter three numbers. The numbers are read in lines 10-12. You
may enter three numbers separated by spaces, then press the
Enter
key, or enter each number
followed by a press of the
Enter
key, as shown in the sample runs of this program.
If you entered an input other than a numeric value, a runtime error would occur. In Chapter
14, you will learn how to handle the exception so that the program can continue to run.
runtime error
Note
Most of the programs in the early chapters of this topic perform three steps: input,
process, and output, called
IPO.
Input is receiving input from the user; process is pro-
ducing results using the input; and output is displaying the results.
IPO
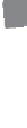
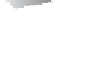



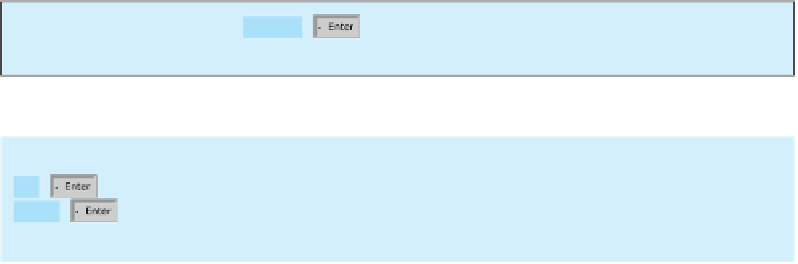

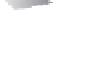












