Java Reference
In-Depth Information
3
4 @Override
/** Paint a Mandelbrot image */
5
protected void
paintComponent(Graphics g) {
6
super
.paintComponent(g);
7
8
for
(
double
x =
-2.0
; x <
2.0
; x +=
0.01
)
9
for
(
double
y =
-2.0
; y <
2.0
; y +=
0.01
) {
10
int
c = count(
new
Complex(x, y));
11
if
(c == COUNT_LIMIT)
12 g.setColor(Color.BLACK);
// c is in a Mandelbrot set
13
else
14 g.setColor(
new
Color(
15 c *
77
%
256
, c *
58
%
256
, c *
159
%
256
));
16
17 g.drawRect((
int
)(x *
100
) +
200
, (
int
)(y *
100
) +
200
,
18
1
,
1
);
// Fill a tiny rectangle with the specified color
19 }
20 }
21
22
/** Return the iteration count */
23
static int
count(Complex c) {
24 Complex z =
new
Complex(
0
,
0
);
// z0
25
26
for
(
int
i =
0
; i < COUNT_LIMIT; i++) {
27 z = z.multiply(z).add(c);
// Get z1, z2, . . .
28
if
(z.abs() >
2
)
return
i;
// The sequence is unbounded
29 }
30
31
return
COUNT_LIMIT;
// Indicate a bounded sequence
32 }
33 }
The
count(Complex c)
method (lines 23-32) computes z1, z2, . . ., z60. If none
of their absolute values exceeds
2
,
we assume
c
is in the Mandelbrot set. Of
course, there could always be an error, but
60 (COUNT_LIMIT)
iterations usually
are enough. Once we find that the sequence is unbounded, the method returns the
(a)
(b)
F
IGURE
15.13
A Mandelbrot image is shown in (a) and a Julia set image is shown in (b).


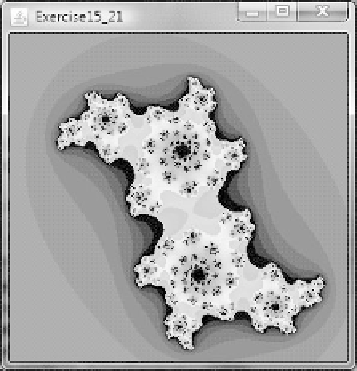
