Java Reference
In-Depth Information
Just like you can read data from a file on your computer, you can read data from a file
on the Web.
Key
Point
In addition to reading data from a local file on a computer or file server, you can also access
data from a file that is on the Web if you know the file's URL (Uniform Resource Locator—
for the file
index.html
located on the Google Web server. When you enter the URL in a Web
browser, the Web server sends the data to your browser, which renders the data graphically.
Figure 14.11 illustrates how this process works.
Client
Server
Web
Browser
Web
Server
Internet
Application
Program
Local files
F
IGURE
14.11
The client retrieves files from a Web server.
For an application program to read data from a URL, you first need to create a
URL
object
using the
java.net.URL
class with this constructor:
public
URL(String spec)
throws
MalformedURLException
For example, the following statement creates a URL object for
http://www.google
1
try
{
2 URL url =
new
URL(
"http://www.google.com/index.html
");
3 }
4
catch
(MalformedURLException ex) {
5 ex.printStackTrace();
6 }
A
MalformedURLException
is thrown if the URL string has a syntax error. For example,
the URL string “
http: www.google.com/index.html
” would cause a
MalformedURLException
runtime error because two slashes (
//
) are required after the colon (
:
). Note that the
http://
prefix is required for the
URL
class to recognize a valid URL. It would be wrong if
you replace line 2 with the following code:
/
URL url =
new
URL(
"www.google.com/index.html"
);
After a
URL
object is created, you can use the
openStream()
method defined in the
URL
class to open an input stream and use this stream to create a
Scanner
object as follows:
Scanner input =
new
Scanner(url.openStream());
Now you can read the data from the input stream just like from a local file. The example in
Listing 14.17 prompts the user to enter a URL and displays the size of the file.
L
ISTING
14.17
ReadFileFromURL.java
1
import
java.util.Scanner;
2




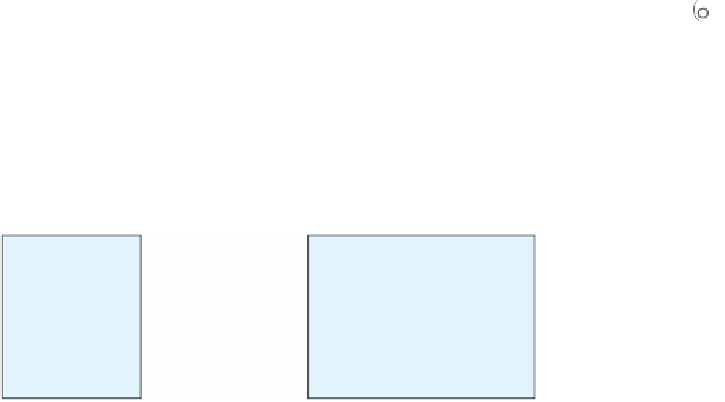


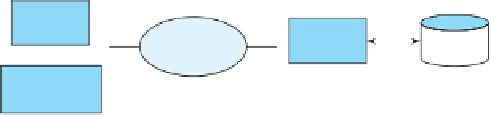


