Java Reference
In-Depth Information
create a
Scanner
9 Scanner input =
new
Scanner(file);
10
11
// Read data from a file
12
while
(input.hasNext()) {
13 String firstName = input.next();
14 String mi = input.next();
15 String lastName = input.next();
16
int
score = input.nextInt();
17 System.out.println(
18 firstName +
""
+ mi +
""
+ lastName +
""
+ score);
19 }
20
21
// Close the file
22 input.close();
23 }
24 }
scores.txt
has next?
read items
John T Smith 90
Eric K Jones 85
close file
java.util.Scanner
+Scanner(source: File)
+Scanner(source: String)
+close()
+hasNext(): boolean
+next(): String
+nextLine(): String
+nextByte(): byte
+nextShort(): short
+nextInt(): int
+nextLong(): long
+nextFloat(): float
+nextDouble(): double
+useDelimiter(pattern: String):
Scanner
Creates a
Scanner
that produces values scanned from the specified file.
Creates a
Scanner
that produces values scanned from the specified string.
Closes this scanner.
Returns true if this scanner has more data to be read.
Returns next token as a string from this scanner.
Returns a line ending with the line separator from this scanner.
Returns next token as a
byte
from this scanner.
Returns next token as a
short
from this scanner.
Returns next token as an
int
from this scanner.
Returns next token as a
long
from this scanner.
Returns next token as a
float
from this scanner.
Returns next token as a
double
from this scanner.
Sets this scanner's delimiting pattern and returns this scanner.
F
IGURE
14.9
The
Scanner
class contains the methods for scanning data.
Note that
new Scanner(String)
creates a
Scanner
for a given string. To create a
Scanner
to read data from a file, you have to use the
java.io.File
class to create an
instance of the
File
using the constructor
new File(filename)
(line 6), and use
new
Scanner(File)
to create a
Scanner
for the file (line 9).
Invoking the constructor
new Scanner(File)
may throw an I/O exception, so the
main
method declares
throws Exception
in line 4.
Each iteration in the
while
loop reads the first name, middle initial, last name, and score
from the text file (lines 12-19). The file is closed in line 22.
It is not necessary to close the input file (line 22), but it is a good practice to do so to
release the resources occupied by the file.
File
class
throws Exception
close file
14.11.3 How Does
Scanner
Work?
The
nextByte()
,
nextShort()
,
nextInt()
,
nextLong()
,
nextFloat()
,
nextDouble()
, and
next()
methods are known as
token-reading methods
, because they
read tokens separated by delimiters. By default, the delimiters are whitespace. You can use the
useDelimiter(String regex)
method to set a new pattern for delimiters.
token-reading method
change delimiter
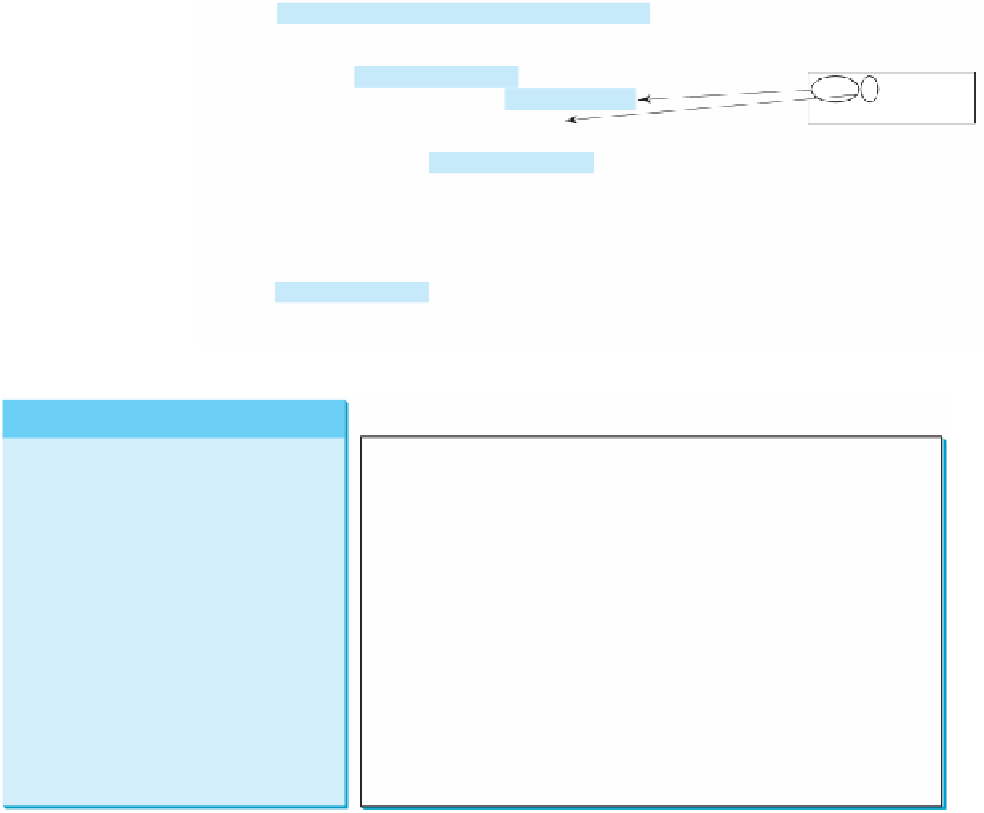

