Java Reference
In-Depth Information
done when an error occurs. The key benefit of exception handling is separating the detection
of an error (done in a called method) from the handling of an error (done in the calling
method).
Many library methods throw exceptions. Listing 14.5 gives an example that handles an
InputMismatchException
when reading an input.
L
ISTING
14.5
InputMismatchExceptionDemo.java
1
import
java.util.*;
2
3
public class
InputMismatchExceptionDemo {
4
public static void
main(String[] args) {
5 Scanner input =
new
Scanner(System.in);
6
create a
Scanner
boolean
continueInput =
true
;
7
8
do
{
9 {
10 System.out.print(
"Enter an integer: "
);
11
12
13
// Display the result
14 System.out.println(
15
try
try
block
int
number = input.nextInt();
If an
InputMismatch
Exception
occurs
"The number entered is "
+ number);
16
17 continueInput =
false
;
18 }
19
20 System.out.println(
"Try again. ("
+
21
"Incorrect input: an integer is required)"
);
22 input.nextLine();
// Discard input
23 }
24 }
while
(continueInput);
25 }
26 }
catch
(InputMismatchException ex) {
catch
block
Enter an integer:
Try again. (Incorrect input: an integer is required)
Enter an integer:
The number entered is 4
3.5
4
When executing
input.nextInt()
(line 11), an
InputMismatchException
occurs if
the input entered is not an integer. Suppose
3.5
is entered. An
InputMismatchException
occurs and the control is transferred to the
catch
block. The statements in the
catch
block
are now executed. The statement
input.nextLine()
in line 22 discards the current input
line so that the user can enter a new line of input. The variable
continueInput
controls the
loop. Its initial value is
true
(line 6), and it is changed to
false
(line 17) when a valid input
is received. Once a valid input is received, there is no need to continue the input.
14.1
What is the advantage of using exception handling?
14.2
Which of the following statements will throw an exception?
✓
✓
Check
Point
System.out.println(
1
/
0
);
System.out.println(
1.0
/
0
);

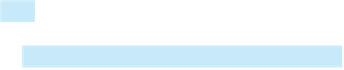
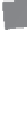
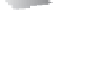




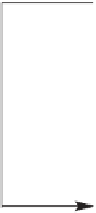




