Java Reference
In-Depth Information
more detailed guidelines, see Supplement I.D, Java Coding Style Guidelines, on the Com-
panion Website.
1.10.1 Appropriate Comments and Comment Styles
Include a summary at the beginning of the program that explains what the program does, its
key features, and any unique techniques it uses. In a long program, you should also include
comments that introduce each major step and explain anything that is difficult to read. It is
important to make comments concise so that they do not crowd the program or make it diffi-
cult to read.
In addition to line comments (beginning with
//
) and block comments (beginning with
/*
), Java supports comments of a special type, referred to as
javadoc comments.
javadoc
comments begin with
/**
and end with
*/
. They can be extracted into an HTML file using
the JDK's
javadoc
command. For more information, see
java.sun.com/j2se/javadoc
.
Use javadoc comments (
/** ... */
) for commenting on an entire class or an entire
method. These comments must precede the class or the method header in order to be extracted
into a javadoc HTML file. For commenting on steps inside a method, use line comments (
//
).
To see an example of a javadoc HTML file, check out
www.cs.armstrong.edu/liang/javadoc/
javadoc comment
1.10.2 Proper Indentation and Spacing
A consistent indentation style makes programs clear and easy to read, debug, and maintain.
Indentation
is used to illustrate the structural relationships between a program's components
or statements. Java can read the program even if all of the statements are on the same long line,
but humans find it easier to read and maintain code that is aligned properly. Indent each sub-
component or statement at least
two
spaces more than the construct within which it is nested.
A single space should be added on both sides of a binary operator, as shown in the follow-
ing statement:
indent code
System.out.println(
3
+
4
*
4
);
Bad style
System.out.println(
3
+
4
*
4
);
Good style
1.10.3 Block Styles
A
block
is a group of statements surrounded by braces. There are two popular styles,
next-line
style and
end-of-line
style, as shown below.
public class
Test
{
public class
Test {
public static void
main(String[] args) {
System.out.println(
"Block Styles"
);
public static void
main(String[] args)
{
}
System.out.println(
"Block Styles"
);
}
}
}
Next-line style
End-of-line style
The next-line style aligns braces vertically and makes programs easy to read, whereas the
end-of-line style saves space and may help avoid some subtle programming errors. Both are
acceptable block styles. The choice depends on personal or organizational preference. You
should use a block style consistently—mixing styles is not recommended. This topic uses the
end-of-line
style to be consistent with the Java API source code.
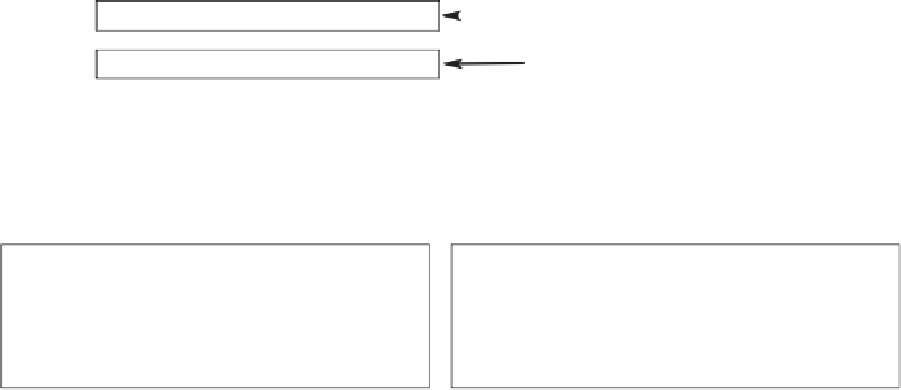