Java Reference
In-Depth Information
4
public class
TestPaintComponent
extends
JFrame {
5
public
TestPaintComponent() {
6 add(
new
NewPanel()
);
create a panel
7 }
8
9
public static void
main(String[] args) {
10 TestPaintComponent frame =
new
TestPaintComponent();
11 frame.setTitle(
"TestPaintComponent"
);
12 frame.setSize(
200
,
100
);
13 frame.setLocationRelativeTo(
null
);
// Center the frame
14 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
15 frame.setVisible(
true
);
16 }
17 }
18
19
20
21
22
23
24
25
26 }
class
NewPanel
extends
JPanel {
new panel class
@Override
override
paintComponent
draw things in the superclass
draw line
draw string
protected void
paintComponent(Graphics g) {
super
.paintComponent(g);
g.drawLine(
0
,
0
,
50
,
50
);
g.drawString(
"Banner"
,
0
,
40
);
}
(0, 0)
(0, 40)
This is a
JPanel
object placed
inside a frame
(50, 50)
F
IGURE
13.4
A line and a string are drawn on a panel.
The
paintComponent
method is automatically invoked to paint graphics when the com-
ponent is first displayed or whenever the component needs to be redisplayed. Invoking
super.paintComponent(g)
(line 22) invokes the
paintComponent
method defined in
the superclass. This is necessary to ensure that the viewing area is cleared before a new draw-
ing is displayed. Line 23 invokes the
drawLine
method to draw a line from (
0
,
0
) to (
50
,
50
).
Line 24 invokes the
drawString
method to draw the string
Banner
at location (
0
,
40
).
All the drawing methods have parameters that specify the locations of the subjects to be
drawn. In Java, all measurements are made in pixels.
The JVM invokes
paintComponent
to draw things on a component. The user should
never invoke
paintComponent
directly. For this reason, the protected visibility is sufficient
for
paintComponent
.
Panels are invisible and are used as small containers that group components to achieve a
desired layout. Another important use of
JPanel
is for drawing. You can draw things on any
Swing GUI component, but normally you should use a
JPanel
as a canvas upon which to
draw things. What happens if you replace
JPanel
with
JLabel
in line 19 as follows?
class
NewPanel
extends
JLabel
{
The program will work, but it is not preferred, because
JLabel
is designed for creating a
label, not for drawing. For consistency, this topic will define a canvas class by subclassing
JPanel
.
extends JPanel
?


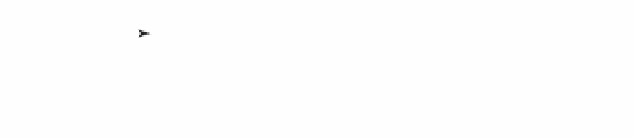
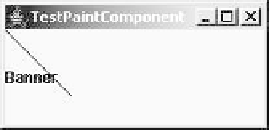





