Java Reference
In-Depth Information
important to be familiar with the methods provided by the
Object
class so that you can use
them in your classes. This section introduces the
toString
method in the
Object
class.
The signature of the
toString()
method is:
toString()
public
String toString()
Invoking
toString()
on an object returns a string that describes the object. By default, it
returns a string consisting of a class name of which the object is an instance, an at sign (
@
),
and the object's memory address in hexadecimal. For example, consider the following code
for the
Loan
class defined in Listing 10.2:
string representation
Loan loan =
new
Loan();
System.out.println(loan.toString());
The output for this code displays something like
Loan@15037e5
. This message is not very
helpful or informative. Usually you should override the
toString
method so that it returns a
descriptive string representation of the object. For example, the
toString
method in the
Object
class was overridden in the
GeometricObject
class in lines 46-49 in Listing 11.1
as follows:
public
String toString() {
return
"created on "
+ dateCreated +
"\ncolor: "
+ color +
" and filled: "
+ filled;
}
Note
You can also pass an object to invoke
System.out.println(object)
or
System.out.print(object)
. This is equivalent to invoking
System.out.println(object.toString())
or
System.out.print(object.toString())
. Thus, you could replace
System.out.println(loan.toString())
with
System.out.println(loan)
.
print object
Polymorphism
means that a variable of a supertype can refer to a subtype object.
Key
Point
The three pillars of object-oriented programming are encapsulation, inheritance, and poly-
morphism. You have already learned the first two. This section introduces polymorphism.
First, let us define two useful terms: subtype and supertype. A class defines a type. A type
defined by a subclass is called a
subtype
, and a type defined by its superclass is called a
supertype
. Therefore, you can say that
Circle
is a subtype of
GeometricObject
and
GeometricObject
is a supertype for
Circle
.
The inheritance relationship enables a subclass to inherit features from its superclass with
additional new features. A subclass is a specialization of its superclass; every instance of a
subclass is also an instance of its superclass, but not vice versa. For example, every circle is a
geometric object, but not every geometric object is a circle. Therefore, you can always pass an
instance of a subclass to a parameter of its superclass type. Consider the code in Listing 11.5.
subtype
supertype
L
ISTING
11.5
PolymorphismDemo.java
1
public class
PolymorphismDemo {
2
/** Main method */
3
public static void
main(String[] args) {



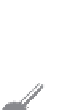
