Java Reference
In-Depth Information
14 System.out.println(s +
" is a palindrome"
);
15
else
16 System.out.println(s +
" is not a palindrome"
);
17 }
18
19
/** Check if a string is a palindrome */
20
public static boolean
isPalindrome(
String s
) {
21
// The index of the first character in the string
22
int
low =
0
;
low index
23
24
// The index of the last character in the string
s.length()
25
int
high =
-
1
;
high index
26
27
while
(low < high) {
28
if
(
s.charAt(low) != s.charAt(high)
)
29
return false
;
// Not a palindrome
30
31 low++;
32 high——;
33 }
34
35
update indices
return true
;
// The string is a palindrome
36 }
37 }
noon
Enter a string:
noon is a palindrome
moon
Enter a string:
moon is not a palindrome
The
nextLine()
method in the
Scanner
class (line 11) reads a line into
s
, and then
isPalindrome(s)
checks whether
s
is a palindrome (line 13).
This section presents a program that converts a hexadecimal number into a decimal
number.
Key
Point
Section 5.7 gives a program that converts a decimal to a hexadecimal. How do you convert a
hex number into a decimal?
Given a hexadecimal number
h
n
h
n
-
1
h
n
-
2
. . .
h
2
h
1
h
0
,
the equivalent decimal value is
16
n
16
n
-
1
16
n
-
2
16
2
16
1
16
0
h
n
*
+
h
n
-
1
*
+
h
n
-
2
*
+
. . .
+
h
2
*
+
h
1
*
+
h
0
*
For example, the hex number
AB8C
is
16
3
16
2
16
1
16
0
10
*
+
11
*
+
8
*
+
12
*
=
43916
Our program will prompt the user to enter a hex number as a string and convert it into a deci-
mal using the following method:
public static int
hexToDecimal(String hex)
A brute-force approach is to convert each hex character into a decimal number, multiply it by
for a hex digit at the
i
's position, and then add all the items together to obtain the equiva-
lent decimal value for the hex number.
16
i


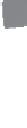







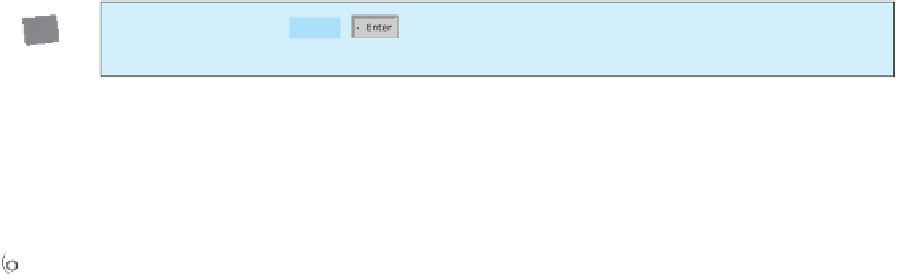
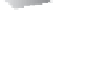













