Java Reference
In-Depth Information
java.lang.String
+indexOf(ch: char): int
Returns the index of the first occurrence of
ch
in the string.
Returns
-1
if not matched.
Returns the index of the first occurrence of
ch
after
fromIndex
in
the string. Returns
-1
if not matched.
Returns the index of the first occurrence of string
s
in this string.
Returns
-1
if not matched.
Returns the index of the first occurrence of string
s
in this string
after
fromIndex
. Returns
-1
if not matched.
Returns the index of the last occurrence of
ch
in the string.
Returns
-1
if not matched.
Returns the index of the last occurrence of
ch
before
fromIndex
in this string. Returns
-1
if not matched.
Returns the index of the last occurrence of string
s
. Returns
-1
if
not matched.
Returns the index of the last occurrence of string
s
before
fromIndex
. Returns
-1
if not matched.
+indexOf(ch: char, fromIndex:
int): int
+indexOf(s: String): int
+indexOf(s: String, fromIndex:
int): int
+lastIndexOf(ch: int): int
+lastIndexOf(ch: int,
fromIndex: int): int
+lastIndexOf(s: String): int
+lastIndexOf(s: String,
fromIndex: int): int
F
IGURE
9.8
The
String
class contains the methods for matching substrings.
For example,
"Welcome to Java".indexOf('W')
returns
0
.
"Welcome to Java".indexOf('o')
indexOf
returns
4
.
"Welcome to Java".indexOf('o', 5)
returns
9
.
"Welcome to Java".indexOf("come")
returns
3
.
"Welcome to Java".indexOf("Java", 5)
returns
11
.
"Welcome to Java".indexOf("java", 5)
returns
-1
.
"Welcome to Java".lastIndexOf('W')
returns
0
.
"Welcome to Java".lastIndexOf('o')
lastIndexOf
returns
9
.
"Welcome to Java".lastIndexOf('o', 5)
returns
4
.
"Welcome to Java".lastIndexOf("come")
returns
3
.
"Welcome to Java".lastIndexOf("Java", 5)
returns
-1
.
"Welcome to Java".lastIndexOf("Java")
returns
11
.
9.2.9 Conversion between Strings and Arrays
Strings are not arrays, but a string can be converted into an array, and vice versa. To convert a
string into an array of characters, use the
toCharArray
method. For example, the following
statement converts the string
Java
to an array.
toCharArray
char
[] chars =
"Java"
.toCharArray();
Thus,
chars[0]
is
J
,
chars[1]
is
a
,
chars[2]
is
v
, and
chars[3]
is
a
.
You can also use the
getChars(int srcBegin, int srcEnd, char[] dst, int
dstBegin)
method to copy a substring of the string from index
srcBegin
to index
srcEnd-1
into a character array
dst
starting from index
dstBegin
. For example, the fol-
lowing code copies a substring
"3720"
in
"CS3720"
from index
2
to index
6-1
into the char-
acter array
dst
starting from index
4
.
getChars
char
[] dst = {
'J'
,
'A'
,
'V'
,
'A'
,
'1'
,
'3'
,
'0'
,
'1'
};
"CS3720"
.getChars(
2
,
6
, dst,
4
);
Thus,
dst
becomes
{'J', 'A', 'V', 'A', '3', '7', '2', '0'}
.
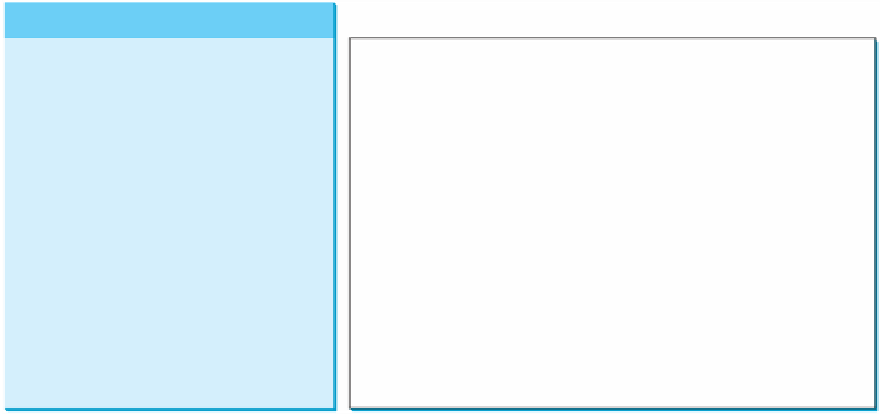