Java Reference
In-Depth Information
The program creates an array of
99 boolean
elements and initializes each element to
false
(line 6). It reads the first number from the file (line 9). The program then repeats the
following operations in a loop:
If the number is not zero, set its corresponding value in array
isCovered
to
true
(line 11);
■
Read the next number (line 12).
■
When the input is
0
, the input ends. The program checks whether all numbers are covered in
lines 16-21 and displays the result in lines 24-27.
The problem is to create a program that will randomly select four cards from a deck of
cards.
Key
Point
Say you want to write a program that will pick four cards at random from a deck of
52
cards.
All the cards can be represented using an array named
deck
, filled with initial values
0
to
51
,
as follows:
int
[] deck =
new int
[
52
];
// Initialize cards
for
(
int
i =
0
; i < deck.length; i++)
deck[i] = i;
Card numbers
0
to
12
,
13
to
25
,
26
to
38
, and
39
to
51
represent 13 Spades, 13 Hearts,
13 Diamonds, and 13 Clubs, respectively, as shown in Figure 6.3.
cardNumber / 13
deter-
mines the suit of the card and
cardNumber % 13
determines the rank of the card, as shown
in Figure 6.4. After shuffling the array
deck
, pick the first four cards from
deck
. The pro-
gram displays the cards from these four card numbers.
deck
[0]
.
.
.
[12]
[13]
.
.
.
[25]
[26]
.
.
.
[38]
[39]
.
.
.
[51]
deck
[0]
[1]
[2]
[3]
[4]
[5]
.
.
.
[25]
[26]
.
.
.
[38]
[39]
.
.
.
[51]
0
.
.
.
12
1
.
.
.
25
2
.
.
.
38
3
.
.
.
51
0
.
.
.
12
13
.
.
.
25
26
.
.
.
38
39
.
.
.
51
6
48
11
24
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
Card number 6 is the
7 (6 % 13 = 6) of
Spades (7 / 13 is 0)
Card number 48 is the
10 (48 % 13 = 9) of
Clubs (48 / 13 is 3)
13 Spades ( )
13 Hearts ( )
Card number 11 is the
Queen (11 % 13 = 11) of
Spades (11 / 13 is 0)
Random shuffle
Card number 24 is the
Queen (24 % 13 = 11) of
Hearts (24 / 13 is 1)
13 Diamonds ( )
13 Clubs ( )
F
IGURE
6.3
52 cards are stored in an array named
deck
.


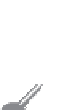


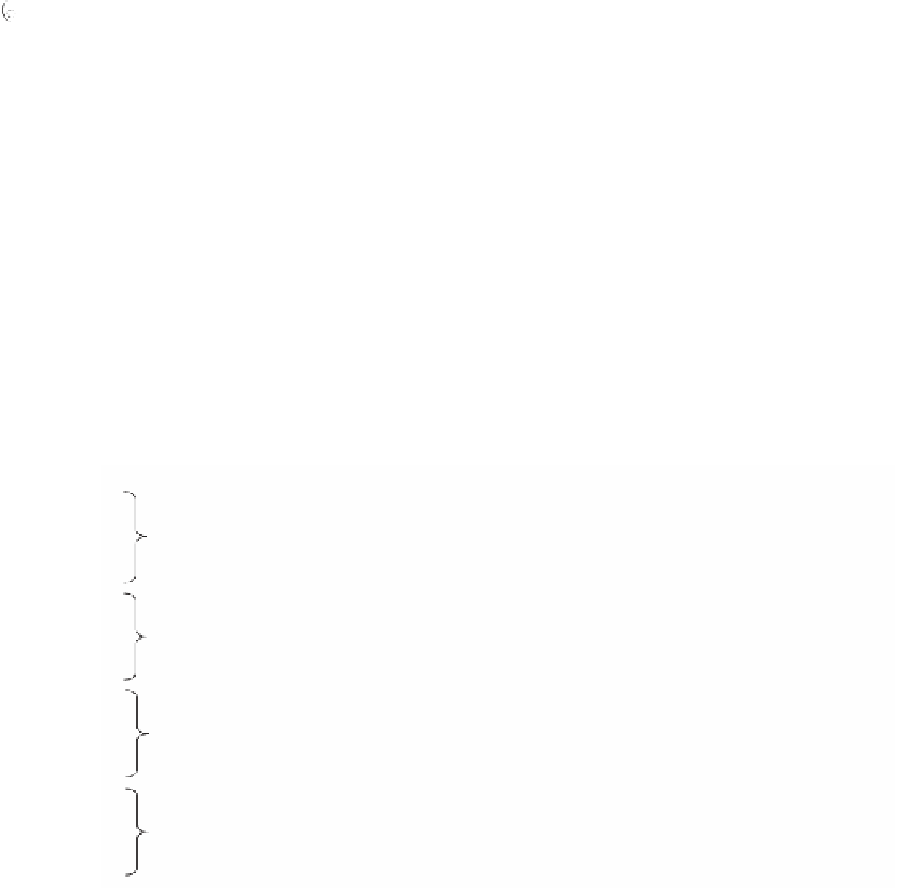







