Java Reference
In-Depth Information
5.18
(
Use the
Math.sqrt
method
) Write a program that prints the following table
using the
sqrt
method in the
Math
class.
Number SquareRoot
0 0.0000
2 1.4142
...
18 4.2426
20 4.4721
*5.19
(
The
MyTriangle
class
) Create a class named
MyTriangle
that contains the
following two methods:
/** Return true if the sum of any two sides is
* greater than the third side. */
public static boolean
isValid(
double
side1,
double
side2,
double
side3)
/** Return the area of the triangle. */
public static double
area(
double
side1,
double
side2,
double
side3)
Write a test program that reads three sides for a triangle and computes the area if
the input is valid. Otherwise, it displays that the input is invalid. The formula for
computing the area of a triangle is given in Programming Exercise 2.15.
5.20
(
Use trigonometric methods
) Print the following table to display the
sin
value and
cos
value of degrees from 0 to 360 with increments of 10 degrees. Round the
value to keep four digits after the decimal point.
Degree
Sin
Cos
0
0.0000
1.0000
10
0.1736
0.9848
...
350
-0.1736
0.9848
360
0.0000
1.0000
*5.21
(Geometry: great circle distance
) The great circle distance is the distance between
two points on the surface of a sphere. Let (x1, y1) and (x2, y2) be the geographical
latitude and longitude of two points. The great circle distance between the two
points can be computed using the following formula:
d
=
radius
*
arccos(sin(
x
1
)
*
sin(
x
2
)
+
cos(
x
1
)
*
cos(
x
2
)
*
cos(
y
1
-
y
2
))
Write a program that prompts the user to enter the latitude and longitude of two
points on the earth in degrees and displays its great circle distance. The average
earth radius is 6,371.01 km. Note that you need to convert the degrees into radians
using the
Math.toRadians
method since the Java trigonometric methods use
radians. The latitude and longitude degrees in the formula are for North and West.
Use negative to indicate South and East degrees. Here is a sample run:
Enter point 1 (la
t
itude and longitude) in degrees:
39.55 -116.25
Enter point 2
(
latitude and longitude) in degrees:
41.5 87.37
The distance between the two points is 10691.79183231593 km
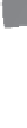
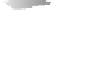




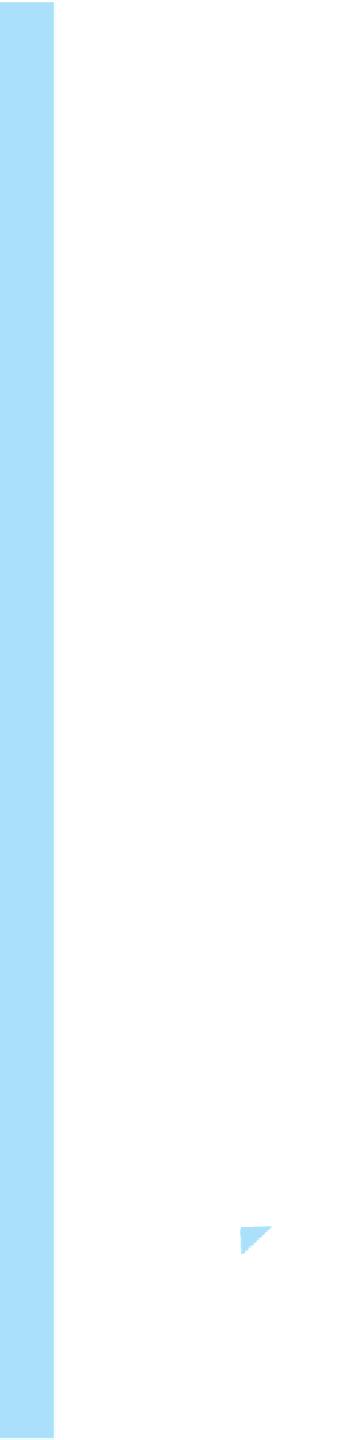


