Java Reference
In-Depth Information
Can you invoke the
max
method with an
int
value and a
double
value, such as
max(2,
2.5)
? If so, which of the
max
methods is invoked? The answer to the first question is yes. The
answer to the second question is that the
max
method for finding the maximum of two
double
values is invoked. The argument value
2
is automatically converted into a
double
value and passed to this method.
You may be wondering why the method
max(double, double)
is not invoked for the
call
max(3, 4)
. Both
max(double, double)
and
max(int, int)
are possible matches
for
max(3, 4)
. The Java compiler finds the most specific method for a method invocation.
Since the method
max(int, int)
is more specific than
max(double, double)
,
max(int, int)
is used to invoke
max(3, 4)
.
Tip
Overloading methods can make programs clearer and more readable. Methods that perform
the same function with different types of parameters should be given the same name.
Note
Overloaded methods must have different parameter lists. You cannot overload methods
based on different modifiers or return types.
Note
Sometimes there are two or more possible matches for an invocation of a method, but
the compiler cannot determine the most specific match. This is referred to as
ambiguous
invocation.
Ambiguous invocation causes a compile error. Consider the following code:
ambiguous invocation
public class
AmbiguousOverloading {
public static void
main(String[] args) {
System.out.println(
max(
1, 2
)
);
}
public static double
max(
int
num1,
double
num2)
{
if
(num1 > num2)
return
num1;
else
return
num2;
}
public static double
max(
double
num1,
int
num2)
{
if
(num1 > num2)
return
num1;
else
return
num2;
}
}
Both
max(int, double)
and
max(double, int)
are possible candidates to match
max(1, 2)
. Because neither is more specific than the other, the invocation is ambigu-
ous, resulting in a compile error.
5.17
✓
✓
What is method overloading? Is it permissible to define two methods that have the
same name but different parameter types? Is it permissible to define two methods in a
class that have identical method names and parameter lists but different return value
types or different modifiers?
Check
Point
5.18
What is wrong in the following program?
public class
Test {
public static void
method(
int
x) {
}
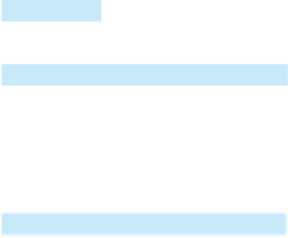



