Java Reference
In-Depth Information
For example, the decimal number
123
is
7B
in hexadecimal. The conversion is done as
follows. Divide
123
by
16
. The remainder is
11
(
B
in hexadecimal) and the quotient is
7
.
Continue to divide
7
by
16
. The remainder is
7
and the quotient is
0
. Therefore
7B
is the hexa-
decimal number for
123
.
0
7
Quotient
16
7
0
7
16
123
112
11
Remainder
h
1
h
0
Listing 5.8 gives a program that prompts the user to enter a decimal number and converts
it into a hex number as a string.
L
ISTING
5.8
Decimal2HexConversion.java
1
import
java.util.Scanner;
2
3
public class
Decimal2HexConversion {
4
/** Main method */
5
public static void
main(String[] args) {
6
// Create a Scanner
7 Scanner input =
new
Scanner(System.in);
8
9
// Prompt the user to enter a decimal integer
10 System.out.print(
"Enter a decimal number: "
);
11
input decimal
int
decimal = input.nextInt();
12
13 System.out.println(
"The hex number for decimal "
+
14 decimal +
" is "
+ decimalToHex(decimal));
15 }
16
17
decimal to hex
/** Convert a decimal to a hex as a string */
18
19 String hex =
""
;
20
21
while
(decimal !=
0
) {
22
int
hexValue = decimal %
16
;
23 hex =
public static
String decimalToHex(
int
decimal) {
toHexChar(hexValue)
+ hex;
24 decimal = decimal /
16
;
25 }
26
27
get a hex char
return
hex;
28 }
29
30
/** Convert an integer to a single hex digit in a character */
31
32
public static char
toHexChar(
int
hexValue) {
if
(hexValue <=
9
&& hexValue >=
0
)
33
return
(
char
)(hexValue +
'0'
)
;
34
else
// hexValue <= 15 && hexValue >= 10
get a letter
35
return
(
char
)(hexValue -
10
+
'A'
)
;
36 }
37 }
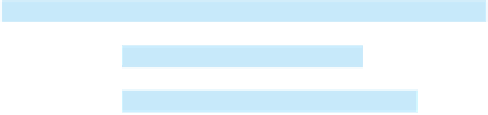


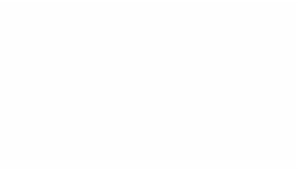
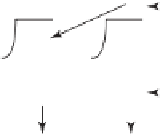
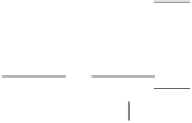