Java Reference
In-Depth Information
public static char
getGrade(
double
score) {
getGrade
method
7
8
if
(score >=
90.0
)
9
return
'A'
;
10
else if
(score >=
80.0
)
11
return
'B'
;
12
else if
(score >=
70.0
)
13
return
'C'
;
14
else if
(score >=
60.0
)
15
return
'D'
;
16
else
17
return
'F'
;
18 }
19 }
The grade is C
The grade is F
The
getGrade
method defined in lines 7-18 returns a character grade based on the numeric
score value. The caller invokes this method in lines 3-4.
The
getGrade
method can be invoked by a caller wherever a character may appear. The
printGrade
method does not return any value, so it must be invoked as a statement.
Note
A
return
statement is not needed for a
void
method, but it can be used for terminat-
ing the method and returning to the method's caller. The syntax is simply
return in void method
return
;
This is not often done, but sometimes it is useful for circumventing the normal flow of
control in a
void
method. For example, the following code has a return statement to
terminate the method when the score is invalid.
public static void
printGrade(
double
score) {
if
(score <
0
|| score >
100
) {
System.out.println(
"Invalid score"
);
return
;
}
if
(score >=
90.0
) {
System.out.println(
'A'
);
}
else if
(score >=
80.0
) {
System.out.println(
'B'
);
}
else if
(score >=
70.0
) {
System.out.println(
'C'
);
}
else if
(score >=
60.0
) {
System.out.println(
'D'
);
}
else
{
System.out.println(
'F'
);
}
}

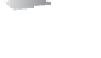







