Java Reference
In-Depth Information
L
ISTING
3.5
ComputeAndInterpretBMI.java
1
import
java.util.Scanner;
2
3
public class
ComputeAndInterpretBMI {
4
public static void
main(String[] args) {
5 Scanner input =
new
Scanner(System.in);
6
7
// Prompt the user to enter weight in pounds
8 System.out.print(
"Enter weight in pounds: "
);
9
input weight
double
weight = input.nextDouble();
10
11
// Prompt the user to enter height in inches
12 System.out.print(
"Enter height in inches: "
);
13
input height
double
height = input.nextDouble();
14
15
final double
KILOGRAMS_PER_POUND =
0.45359237
;
// Constant
16
final double
METERS_PER_INCH =
0.0254
;
// Constant
17
18
// Compute BMI
19
double
weightInKilograms = weight * KILOGRAMS_PER_POUND;
20
double
heightInMeters = height * METERS_PER_INCH;
21
double
bmi = weightInKilograms /
22 (heightInMeters * heightInMeters);
23
24
// Display result
25 System.out.println(
"BMI is "
+ bmi);
26
if
(bmi <
18.5
)
27 System.out.println(
"Underweight"
);
28
else if
(bmi <
25
)
29 System.out.println(
"Normal"
);
30
else if
(bmi <
30
)
31 System.out.println(
"Overweight"
);
32
else
33 System.out.println(
"Obese"
);
34 }
35 }
compute
bmi
display output
Enter weight in pounds:
Enter height in inches:
BMI is 20.948603801493316
Normal
146
70
line#
weight
height weightInKilograms heightInMeters bmi
output
9
146
13
70
19
66.22448602
20
1.778
21
20.9486
25
BMI is
20.95
31
Normal

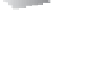


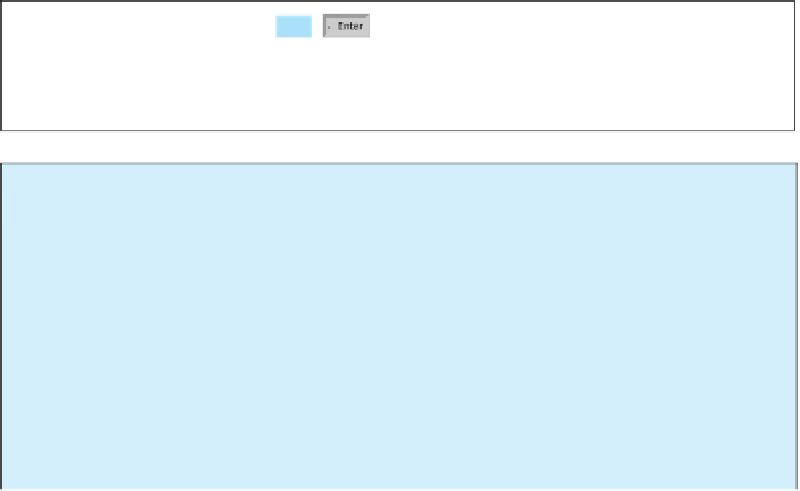








