Game Development Reference
In-Depth Information
void Apply(unsigned int flags, math::rectangle& from, const math::rectangle& to)
{
if ( IsSet(flags, Top) )
{
from.Top() = m_to.Top();
}
else
if ( IsSet(flags, Bottom) )
{
from.Top() = to.Bottom() - from.Height();
}
if ( IsSet(flags, Left) )
{
from.Left() = m_to.Left();
}
else
if ( IsSet(flags, Right) )
{
auto offset = to.Right() - from.Left();
from.Left() += (offset - from.Width());
}
if ( IsSet(flags, Center) )
{
from.Left() = m_to.Left() + (m_to.Width()/2) - (from.Width()/2);
}
if ( IsSet(flags, Middle) )
{
from.Top() = m_to.Top() + (m_to.Height()/2) - (from.Height()/4);
}
}
To align multiple rectangles to a fixed rectangle we can call the Apply function to a refer-
ence to a list of rectangles.
void Apply(unsigned int flags, std::list<math::rectangle>& from, const math::rectangle& to)
{
for ( auto& rectangle : from )
{
Apply(flags, rectangle, to);
}
}
The goal of any helper class such as the align class is to provide an easy way to do actions
that we may want to do often, and to do this, we must design the class to provide the fa-
cilities we need in an intuitive, unobtrusive way. The align class implements a constructor
that allows us to do an immediate alignment in a single line.
align a(floatingRectangle, fixedRectangle, ui::align::Center | ui::align::Middle);
This single line of code will modify
floatingRectangle
to be aligned vertically and hori-
zontally with
fixedRectangle
. However, there may be situations in which we may wish to
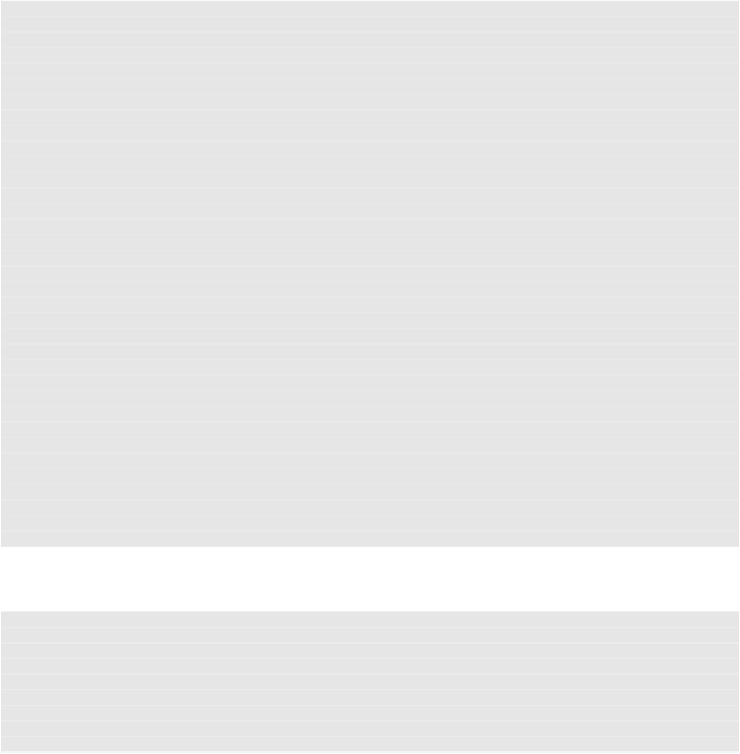



