Game Development Reference
In-Depth Information
{
public:
point GetPointOnEllipse(float angle);
point GetPointOnEllipse(const vector2& direction);
void GetPoints(int count, std::vector<point>& points);
private:
vector2 m_axes; // the semi axes
float m_angle; // angle of the ellipse
vector2 m_center;
};
Get Point on Ellipse
When using an ellipse as a means to constrain some element, it's useful to request a point
that lies directly on the ellipse given an
α
angle.
vector2 GetPointOnEllipse(float angle)
{
float sinAlpha = sin(angle);
float cosAlpha = cos(angle);
float beta = -m_angle;
float sinBeta = sin(beta);
float cosBeta = cos(beta);
float X = (m_axes.x() * cosAlpha * cosBeta - m_axes.y() * sinAlpha * sinBeta);
float Y = (m_axes.x() * cosAlpha * sinBeta + m_axes.y() * sinAlpha * cosBeta);
return m_center + vector2(X,Y);
}
Often in graphics and gameplay systems we use vectors to represent directions rather than
working with angles, it's useful to provide a function that given a direction vector will also
return a point on the ellipse.
point GetPointOnEllipse(const vector2& direction)
{
float alpha = -atan2(direction.x(), direction.y()) + HalfPi;
return GetPointOnEllipse(alpha);
}
Get Point in Ellipse
Another useful function will let us query a point within the ellipse, but constrained by it,
wepassapointtotheellipse,ifthepointiswithintheellipse'sboundarieswereturnit,oth-
erwise we will return the point on the ellipse. This will become useful when we implement
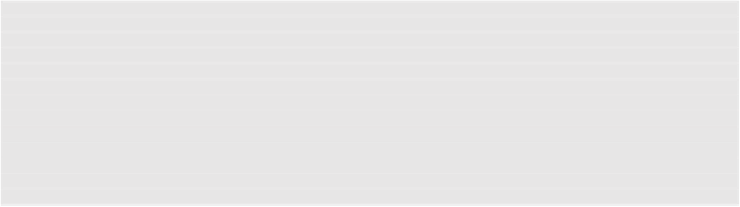
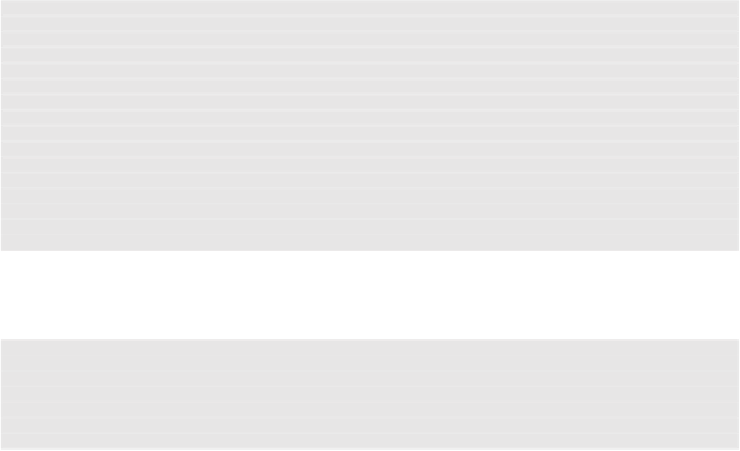


