Game Development Reference
In-Depth Information
late a ray starting at the screen position, projected into world space in a direction into the
world,withthispointanddirectionwecanbuildaray,araythatwethenusetoqueryother
systems for information that we may be interested in, such as, has the player selected an
object? If we find that we have indeed intersected our ray with an object or multiple ob-
jects in the game world. The system we queried with the ray should return the world space
position of the objects it has intersected with, we can then use this information to project
information back onto the screen.
Typically the ray is sent to the physics system which is responsible for detecting any colli-
sions between the ray and any of the objects in the physical representation of the world.
auto p0 = viewport.Unproject(vector3(mouse.x(), mouse.y(), 0.f), camera.View(), camera.Projection(), matrix::Identity);
auto p1 = viewport.Unproject(vector3(mouse.x(), mouse.y(), 1.f), camera.View(), camera.Projection(), matrix::Identity);
auto position = p0;
auto direction = p1 - p0;
direction.Normalize();
QueryResult qr = PhysicsSystem()->Query(ray(position, direction));
if ( qr.Contacts().size() > 0 )
{
for (auto contact : qr.Contacts())
{
// Our ray has intersected with the physical representation of an object
// the contact typically will have a reference or pointer to the game object
// that we have touched.
}
}
This example shows how a ray can be constructed by a mouse press in screen space. We
Unproject
the mouse coordinates at the near and far planes, we use these world space pos-
itions to determine a direction vector. We can then use the world space position and direc-
tion vector to create a ray which we pass into our physics system. If there are any intersec-
tions with game objects we will know as a result of this query. This code will undoubtedly
vary depending on the game engine, some engines may call the
Query
function by some
other name such as
RayCast
or
LineProbe
, more advanced engines will likely provide an
asynchronous method for receiving the results which will change the structure of the code,
generally the concept will be the same.
1.4.1.4Triangles
Triangles are one of the most important rendering primitive, most video hardware expects
data to be organized into triangles prior to rendering, some platforms and frameworks also
use quads, but triangles are more commonly used.
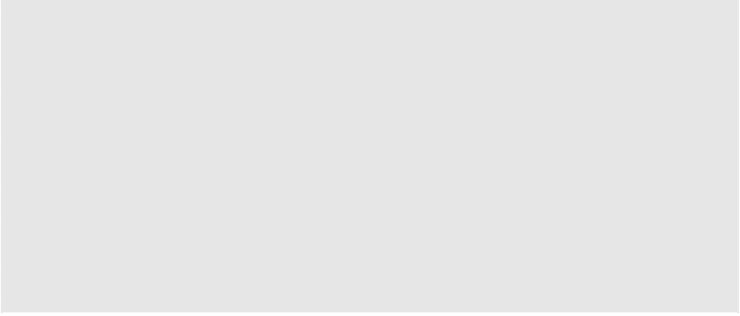


