Game Development Reference
In-Depth Information
saw in the Linear Algebra section, a vector is an entity that represents both a direction and
a magnitude, while a point is a fixed position in space. If you keep this in mind, you can
see how using vectors to represent points may lead to ambiguous functions.
// Ambiguous function that uses vectors to represent points, we rely on the named argument to know that this function expects two positions
in space.
void DrawLine(const vector& pointA, const vector& pointB)
{
. . .
}
// Non-ambigous function declaration clearly states that this function expects two points or positions in space to draw a line.
void DrawLine(const point& a, const point& b)
{
. . .
}
// This function shows that it expects to draw a line from the provided point, in the direction of the vector, using the direction vector's mag-
nitude to determine the length of the line.
void DrawLine(const point& origin, const vector& direction)
{
. . .
}
That said, it may be the case that the software architecture you are using does not provide
a point representation and uses a vectors, in this case, do your best to make it clear to the
caller of a function what parameters are expected and what the function's result will be.
1.4.1.2Lines
We consider a line to be the collection of all the points within two extreme points, in some
cases a line may have one point and extend to infinity. We will not go into much detail
regarding line rasterization as most modern graphics hardware and software APIs already
provide efficient line rasterization features. Instead we will focus on the use of lines for
user interfaces, there are many uses for lines that may not always be necessary for a ship-
ping product, but that are great tools during development and debugging. Often during de-
velopment, we may need to display more information about the world, such as the surface
normals of a geometrical model, or the velocity of an object or sprite, the trajectory of a
projectile, there are countless situations in which drawing lines comes in handy.
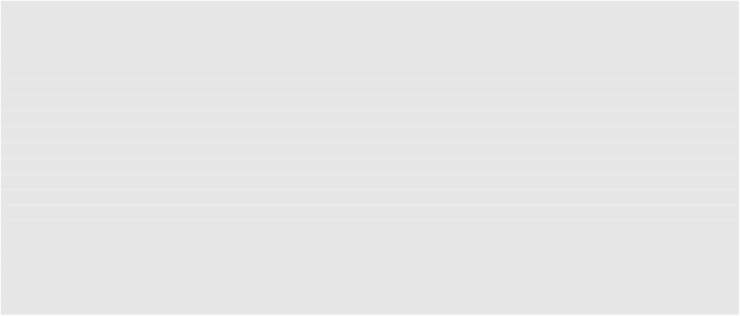


