Game Development Reference
In-Depth Information
When calculating the vertices along the longitude we will at some point reach the end,
when this happens we will wrap around and grab the first vertex to close the ring. The in-
dices to the two triangles are given by:
As a convenience we can use an STL vector to store the indices that we can later use to
build the index buffer.
unsigned short stride = horizontalSegments + 1;
for (unsigned short i = 0; i < verticalSegments; ++i)
{
for (unsigned short j = 0; j <= horizontalSegments; ++j)
{
const unsigned short i1 = i + 1;
const unsigned short j1 = (j + 1) % stride;
const unsigned short ip = i * stride;
const unsigned short np = i1 * stride;
indices.push_back(ip + j);
indices.push_back(np + j);
indices.push_back(ip + j1);
indices.push_back(ip + j1);
indices.push_back(np + j);
indices.push_back(np + j1);
}
}
3.12.5 Matrix Axes
During development it is often very useful to render the transformation matrix for any ob-
ject in the game world. Typically the axes are drawn using the same convention used for
colors, RGB, for R we render the
x
axis red, for G we render the y axis green and for B
we render the
z
axis blue. We render the matrix axes using line primitives, and because we
want to put our newfound knowledge to good use, we will cap each axis' with a cone to
make an arrow.
The goal is that given a transformation matrix, we will draw its basis vectors.

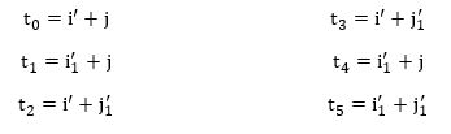


