Game Development Reference
In-Depth Information
15 - Reversi
The
getPlayerMove()
function is called to let the player type in the coordinates of
their next move (and check if the move is valid). The player can also type in
'hints'
to
turn hints mode on (if it is off) or off (if it is on). The player can also type in
'quit'
to
quit the game.
The
DIGITS1TO8
constant variable is the list
['1', '2', '3', '4', '5',
'6', '7', '8']
. We create this constant because it is easier type
DIGITS1TO8
than
the entire list.
192. while True:
193. print('Enter your move, or type quit to end the
game, or hints to turn off/on hints.')
194. move = input().lower()
195. if move == 'quit':
196. return 'quit'
197. if move == 'hints':
198. return 'hints'
The
while
loop will keep looping until the player has typed in a valid move. First we
check if the player wants to quit or toggle hints mode, and return the string
'quit'
or
'hints'
. We use the
lower()
method on the string returned by
input()
so the player
can type
'HINTS'
or
'Quit'
but still have the command understood by our game.
The code that calls
getPlayerMove()
will handle what to do if the player wants to
quit or toggle hints mode.
200. if len(move) == 2 and move[0] in DIGITS1TO8 and
move[1] in DIGITS1TO8:
201. x = int(move[0]) - 1
202. y = int(move[1]) - 1
203. if isValidMove(board, playerTile, x, y) ==
False:
204. continue
205. else:
206. break
Our game is expecting that the player would have typed in the XY coordinates of their
move as two numbers without anything in between them. The
if
statement first checks
that the size of the string the player typed in is
2
. After that, the
if
statement also checks
that both
move[0]
(the first character in the string) and
move[1]
(the second character
in the string) are strings that exist in
DIGITS1TO8
, which we defined at the beginning of
the function.
Remember that our game board data structures have indexes from 0 to 7, not 1 to 8. We
show 1 to 8 when we print the board using
drawBoard()
because people are used to
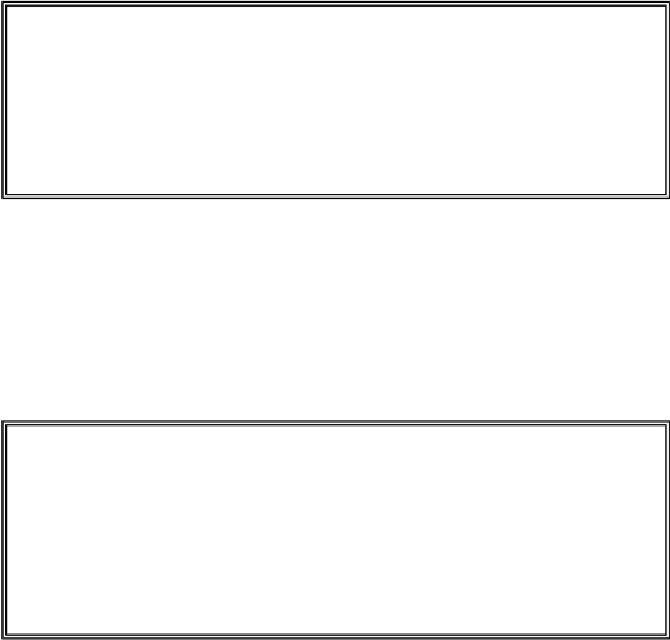