Game Development Reference
In-Depth Information
But if the first space does have the other player's tile, then we should keep proceeding in
that direction until we reach on of our own tiles. If we move off of the board, then we
should continue back to the
for
statement to try the next direction.
69. while board[x][y] == otherTile:
70. x += xdirection
71. y += ydirection
72. if not isOnBoard(x, y): # break out of
while loop, then continue in for loop
73. break
74. if not isOnBoard(x, y):
75. continue
The
while
loop on line 69 ensures that
x
and
y
keep going in the current direction as
long as we keep seeing a trail of the other player's tiles. If
x
and
y
move off of the board,
we break out of the
for
loop and the flow of execution moves to line 74. What we really
want to do is break out of the
while
loop but continue in the
for
loop. But if we put a
continue
statement on line 73, that would only continue to the
while
loop on line 69.
Instead, we recheck
not isOnBoard(x, y)
on line 74 and then continue from
there, which goes to the next direction in the
for
statement. It is important to know that
break
and
continue
will only break or continue in the loop they are called from, and
not an outer loop that contain the loop they are called from.
Finding Out if There are Pieces to Flip Over
76. if board[x][y] == tile:
77. # There are pieces to flip over. Go in
the reverse direction until we reach the original space,
noting all the tiles along the way.
78. while True:
79. x -= xdirection
80. y -= ydirection
81. if x == xstart and y == ystart:
82. break
83. tilesToFlip.append([x, y])
If the
while
loop on line 69 stopped looping because the condition was
False
, then
we have found a space on the board that holds our own tile or a blank space. Line 76
checks if this space on the board holds one of our tiles. If it does, then we have found a
valid move. We start a new
while
loop, this time subtracting
x
and
y
to move them in the
opposite direction they were originally going. We note each space between our tiles on the
board by appending the space to the
tilesToFlip
list.
We break out of the
while
loop once
x
and
y
have returned to the original position
(which was still stored in
xstart
and
ystart
).
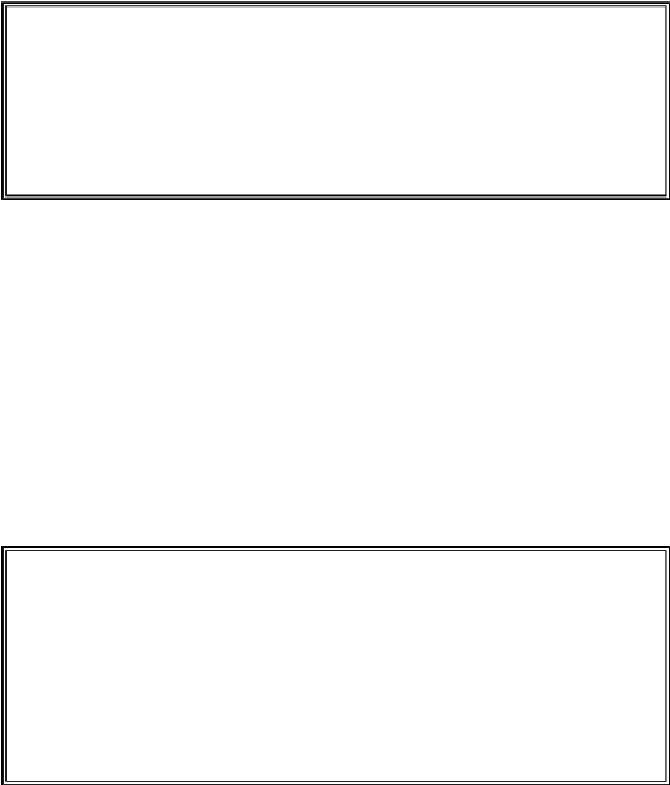